vscode Plugin for LeetCode
About the Plugin and This Site
The solutions and explanations in the plugin are the same as those on this site. The plugin is an auxiliary tool to help you study the content here, but it is not required. Please choose to install it according to your own needs.
Some readers prefer not to practice problems on web pages, and think it's more convenient to write and debug code in an editor or IDE. Therefore, I have developed and maintained plugins for various platforms to meet this need, allowing readers to view problem explanations, visualizations, and problem sets from this site within the plugin.
The vscode plugin allows you to practice LeetCode problems directly in the vscode editor, with support for line-by-line debugging. You can directly view explanations and visualizations from this site, and it also integrates the Quick Mastery Directory and Beginner Directory problem lists for easy review:
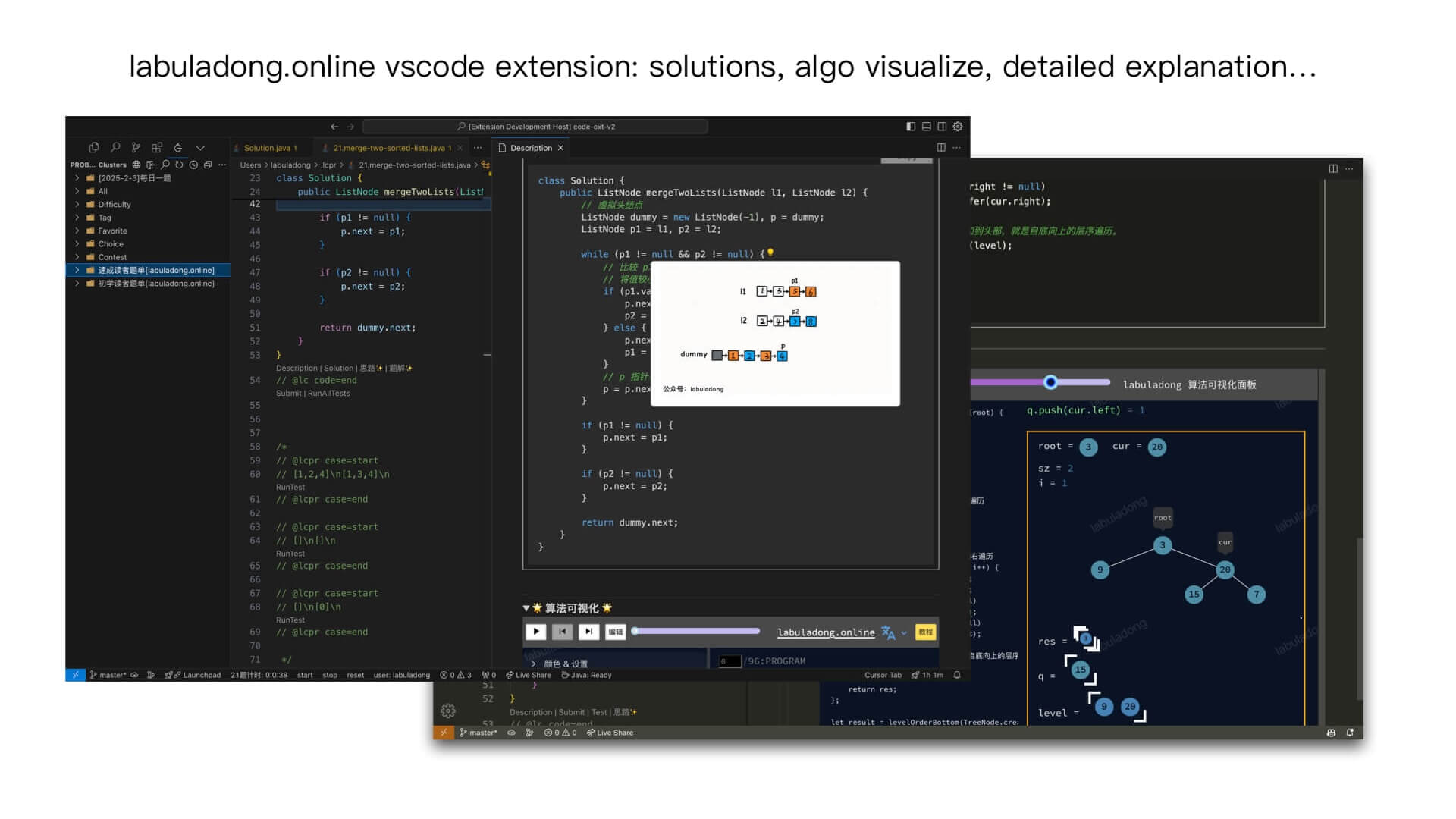
This plugin is based on the following two open-source vscode plugins:
https://github.com/LeetCode-OpenSource/vscode-leetcode
https://github.com/ccagml/leetcode-extension
Each of these plugins has its own advantages and disadvantages. I have tried to combine their strengths and added some useful extra features. Here is a brief introduction.
Installation
Search for the keyword "labuladong" in the vscode plugin marketplace to find the plugin, then click install:
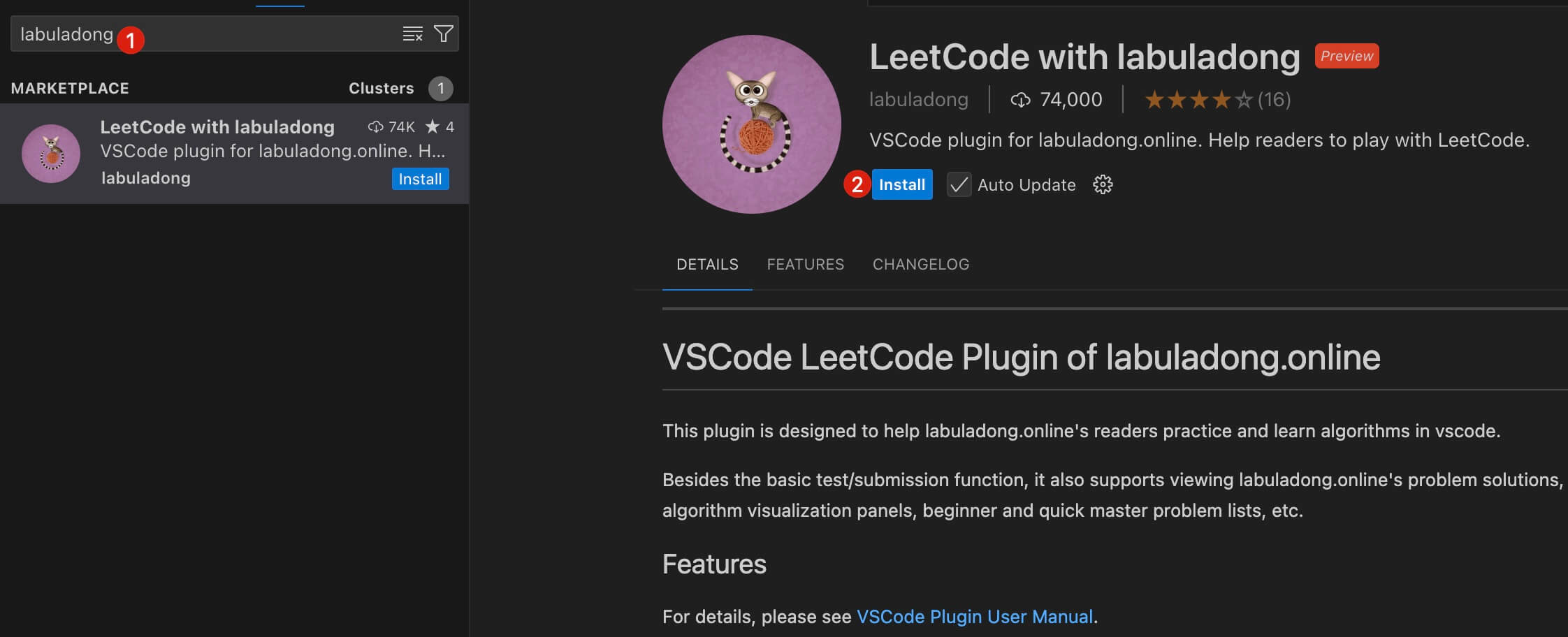
If you can't find it, you can install it from the vscode marketplace website:
https://marketplace.visualstudio.com/items?itemName=labuladong.leetcode-helper
Feature Demo
Problem Solving Helper
All the problem explanations on this site are built into the plugin. In the problem list, questions marked with ✨ have explanations. You can see a short idea or jump to the website for a full explanation:
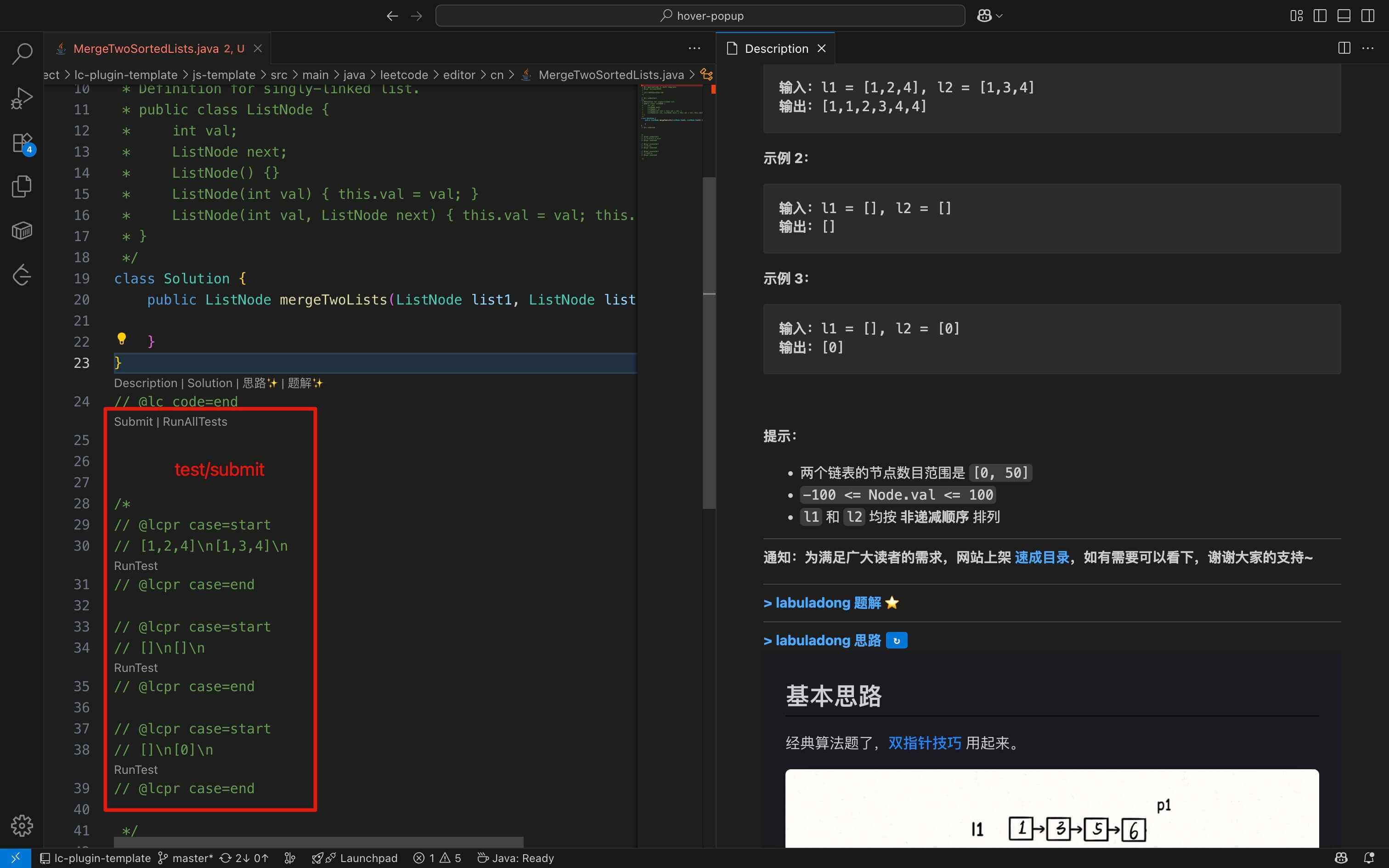
All features of the site are also supported in the plugin, including image notes and algorithm visualization:
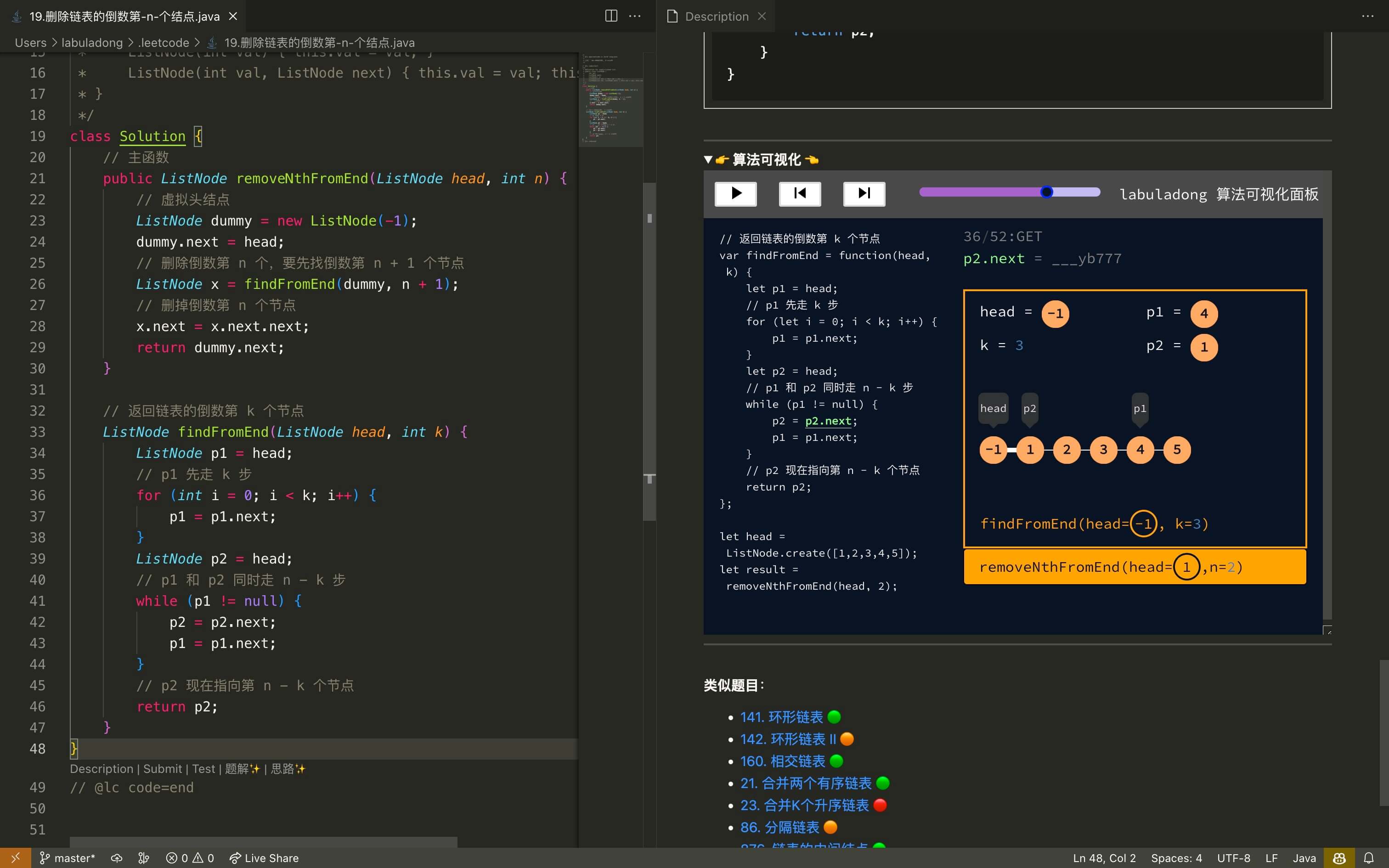
Switch Between Chinese and English
This plugin supports logging in and solving problems on both leetcode.cn (Chinese) and leetcode.com (English).
By default, the explanations, ideas, and visualization panels in the plugin are in Chinese. You can search for the setting keyword labuladongLanguage
in the vscode settings page to switch to English.
Log in to LeetCode
Select a Login Platform
Click the plugin icon on the sidebar and then click the login icon. You will be guided to choose to log in to the Chinese LeetCode or the English LeetCode:
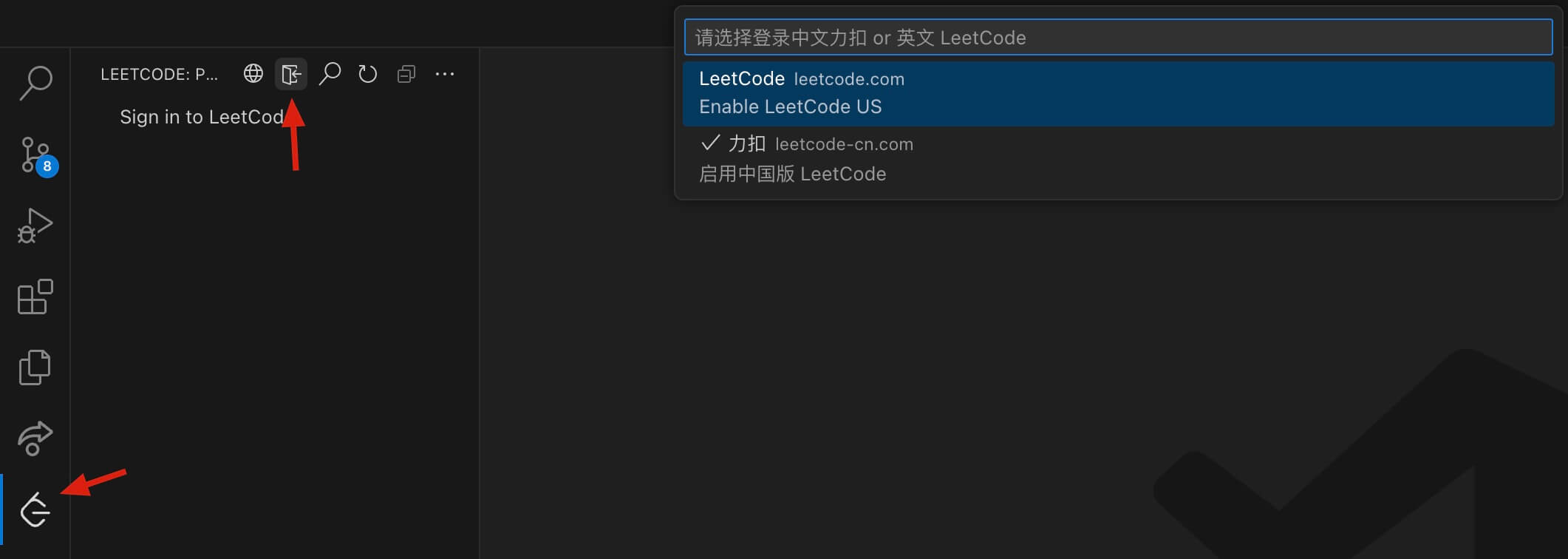
Log in Using a cURL Command
Follow the prompts to enter the cURL command for LeetCode login, and you can complete the login process.
Here is how to get the cURL command:
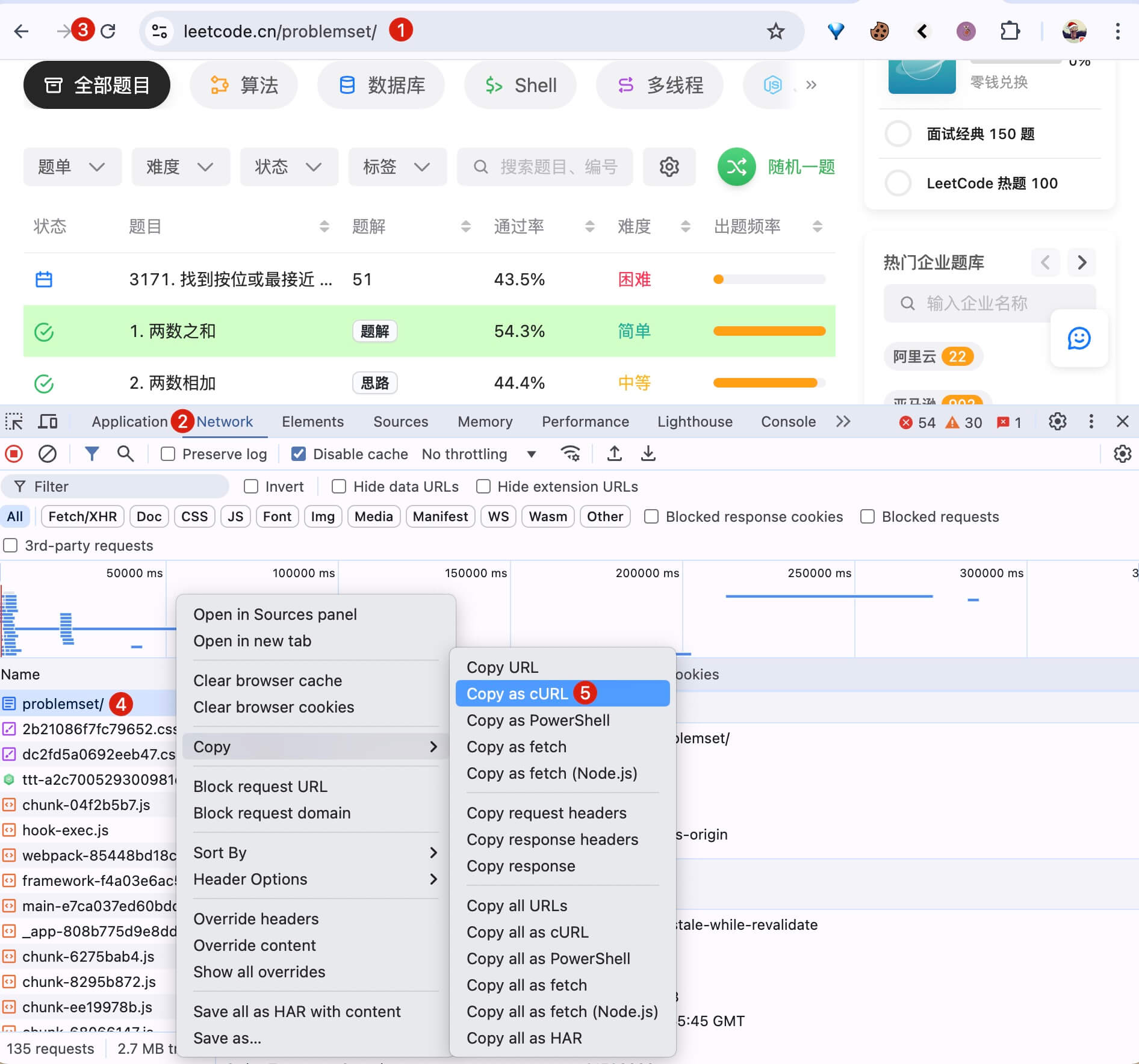
1️⃣ Open the official website https://leetcode.cn/problemset/ (international version at https://leetcode.com/problemset/) and ensure you are logged into your account on the webpage.
2️⃣ Open the browser developer tools (F12 for Chrome/Edge), then click the Network tab.
3️⃣ ~ 4️⃣ Refresh the page, select the first network request, right-click it, and choose Copy
-> Copy as cURL
. For Chrome on Windows, you may need to select Copy
-> Copy as cURL (bash)
.
Login Error?
If you encounter a login error, it is likely that there is an issue with the cURL command from the browser. Check your copied cURL command. It should look like this, containing values for csrftoken=xxx
and LEETCODE_SESSION=xxx
:
curl 'https://leetcode.cn/problemset/' \
-H 'Cookie: ... ; csrftoken=xxx; LEETCODE_SESSION=xxx'; ... \
-H 'Pragma: no-cache' \
-H 'Sec-Fetch-Dest: document' \
...
You can also try pasting the copied cURL command into a terminal to see if it works properly.
Various issues may occur with non-mainstream browsers; it is recommended to use Chrome/Edge for these operations.
Unlocking Solution Ideas/Problem Lists on This Site (Optional)
Note
All users can freely use the plugin’s problem-solving features and access some of the solution ideas for problems on this site. To unlock all solution ideas, algorithm visualizations, and problem lists within the plugin, you need to subscribe to the site.
If you have already subscribed, please follow the steps below to unlock all solutions and ideas in the plugin.
Step 1: Install and Start the Plugin
Install the plugin and log in with your LeetCode account as described above. Make sure the plugin works properly.
Note: After installing the plugin, you will see a LeetCode icon on the left sidebar. Click this icon first to let the plugin finish loading before proceeding. Otherwise, you may encounter command execution failures.
Step 2: Get the Site Cookie
Click the button below to copy the cookie required for logging into the plugin:
Step 3: Enter the Cookie in VS Code
Go to the settings page in VS Code, search for the keyword sitecookie
, and paste the copied cookie string into the input box:
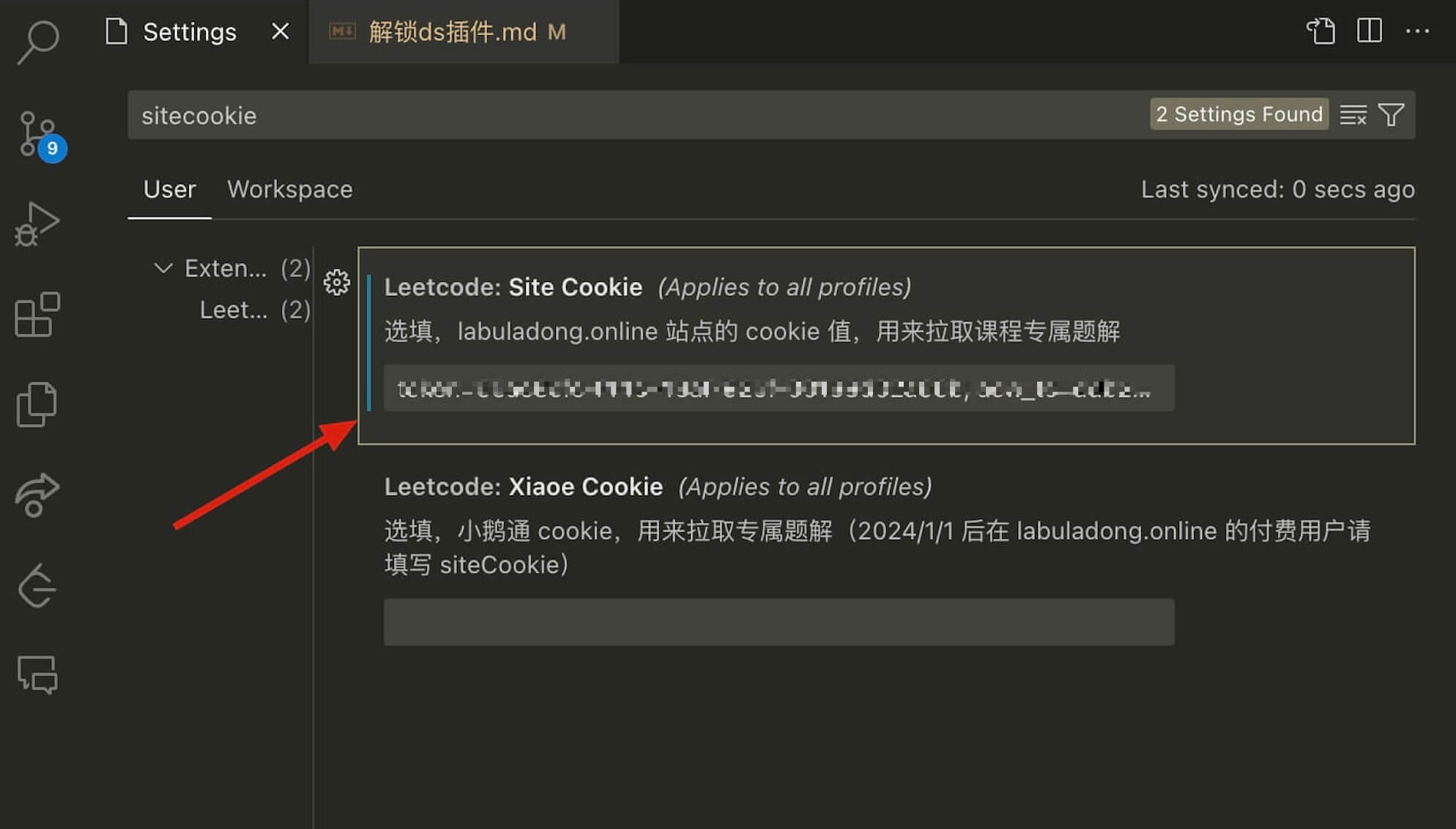
Step 4: Manually Refresh Data
In VS Code, press the F1
key to open the command palette, and enter the keyword labuladong
. You will see an option called Refresh labuladong.online data manually
:

Click or press Enter to refresh. After a few seconds, a notification should confirm that the site subscription data was successfully retrieved:
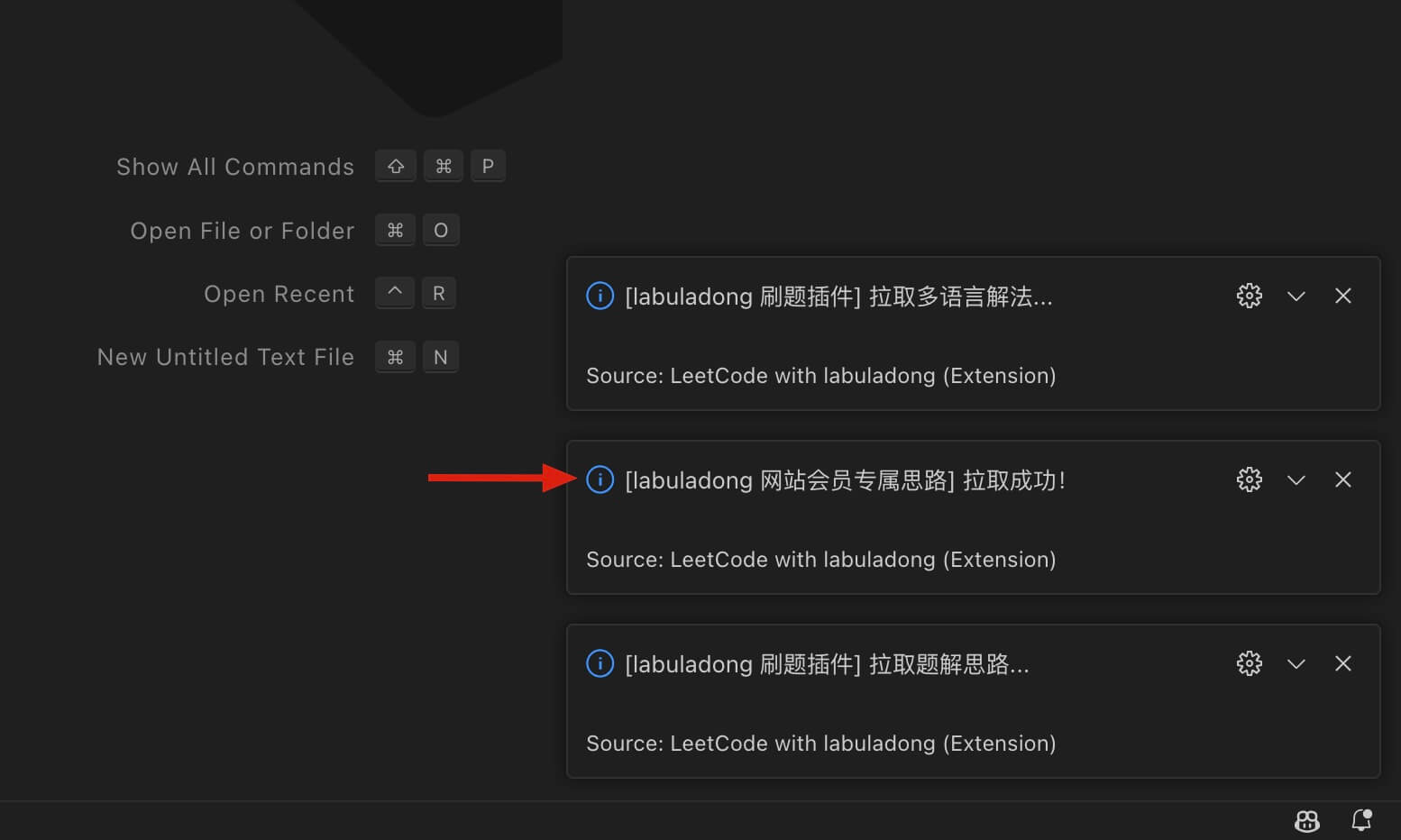
Now, you can view all solution ideas and problem lists from this site directly in VS Code.
Local Debugging of Algorithm Code (Optional)
Note
This part is optional, mainly for readers who want to run and debug code with breakpoints on their own computer:
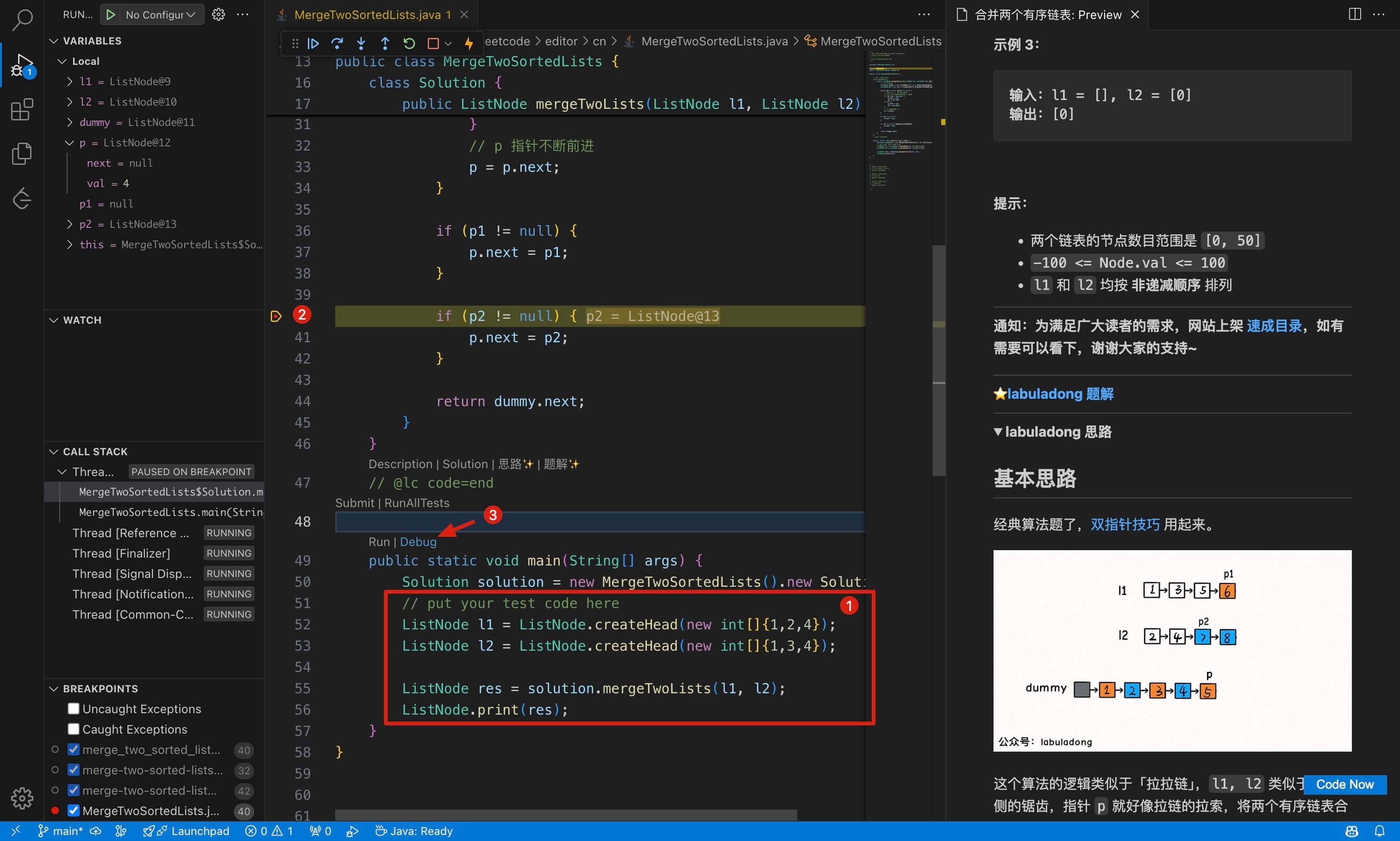
If you do not set this up, the experience is the same as solving problems on the LeetCode website. The code will be submitted to the LeetCode API for testing and judging (you might see type errors in VSCode, but they do not affect submission and can be ignored):
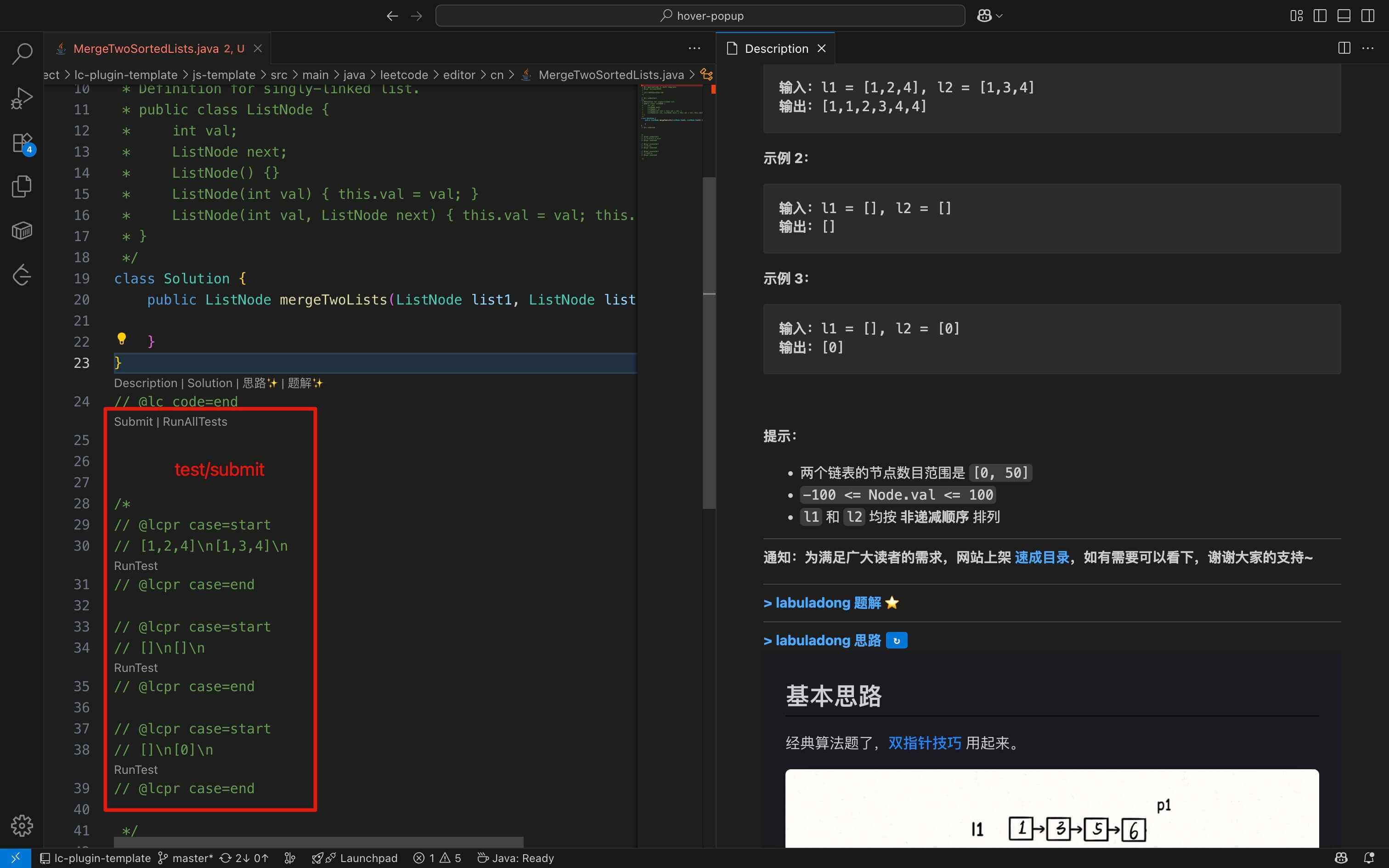
The local debugging feature depends on your own development environment, it is not related to the plugin. If you still see errors after following this guide, please check the error message to fix the problem.
The code for each problem is actually a normal code file, so in theory you can debug and run it locally. But there are a few issues:
LeetCode has some built-in types, such as
ListNode
andTreeNode
. Also, test cases for the problems are given as arrays. You need to implement these structures yourself and convert the input data.You need to do some extra setup for different programming languages, so that your code editor can recognize the project structure. Otherwise, you might not be able to run the code locally or use auto-complete.
To solve these problems, I created a solution. If you follow the steps below, you can run LeetCode problems locally. Currently, Java, C++, Python, Golang, and JavaScript are supported.
Step 1: Clone the Code Template
I have uploaded the configured template project to Github. You can clone it to your computer:
git clone https://github.com/labuladong/lc-plugin-template.git
# If you are in Mainland China, you can download from Gitee:
git clone https://gitee.com/labuladong/lc-plugin-template.git
If you find any problems or have ideas for improvement while using it, feel free to submit a PR or Issue.
Step 2: Set the Code File Path
Search for the keyword labuladong file path
in the VSCode settings page. Click Edit in settings.json
, and set it like this:
"labuladong-leetcode.filePath": {
"python": {
"folder": "leetcode/editor/${endpointType}",
"filename": "${kebab-case-name}.${ext}"
},
"python3": {
"folder": "leetcode/editor/${endpointType}",
"filename": "${kebab-case-name}.${ext}"
},
"javascript": {
"folder": "leetcode/editor/${endpointType}",
"filename": "${kebab-case-name}.${ext}"
},
"cpp": {
"folder": "leetcode/editor/${endpointType}",
"filename": "${kebab-case-name}.${ext}"
},
"golang": {
"folder": "leetcode/editor/${endpointType}",
"filename": "${snake_case_name}_test.${ext}"
},
"java": {
"folder": "src/main/java/leetcode/editor/${endpointType}",
"filename": "${PascalCaseName}.${ext}"
},
"default": {
"folder": "",
"filename": "${id}.${kebab-case-name}.${ext}"
}
},
Step 3: Configure the workspace folder
for Programming Language
Search for the keyword labuladong language
in the settings page of vscode to configure the programming language you use for practice.
Search for the keyword labuladong workspace folder
in the settings page of vscode and configure the working directory according to the programming language you use:
/<your>/<path>/<to>/lc-plugin-template/<your-language>-template/
Here, /<your>/<path>/<to>/
is the path where lc-plugin-template
is cloned locally; the value of <your-language>-template
depends on the programming language you set for practice:
- For Java, configure
java-template
- For C++, configure
cpp-template
- For Python, configure
python-template
- For Golang, configure
go-template
- For JavaScript, configure
js-template
Tip
The path separator for Windows computers is \
instead of /
, and the folder path looks like this:
C:\<your>\<path>\<to>\lc-plugin-template\<your-language>-template\
Please modify according to your actual situation.
Step 3: Configure Code Templates
In the settings page of VS Code, search for the keyword labuladong custom code template
to configure code templates. Once configured, you can locally debug algorithm code.
The configuration I provide only imports the basic standard library. If you wish to import other libraries or have more customized needs, you can modify the code template yourself.
Below is an introduction for different languages.
Java Configuration Method
The configuration for Java code templates is as follows:
package leetcode.editor.${question.endpointType};
import java.util.*;
import leetcode.editor.common.*;
public class $!velocityTool.camelCaseName(${question.titleSlug}) {
${question.codeWithIndent(4)}
public static void main(String[] args) {
#set($className = $!velocityTool.camelCaseName(${question.titleSlug}))
Solution solution = new ${className}().new Solution();
// put your test code here
}
}
After configuration is complete, open the LeetCode problem to write algorithm code, set breakpoints, and write test cases in the main
function. Finally, click the Debug
button above the main
function to debug the code line by line:
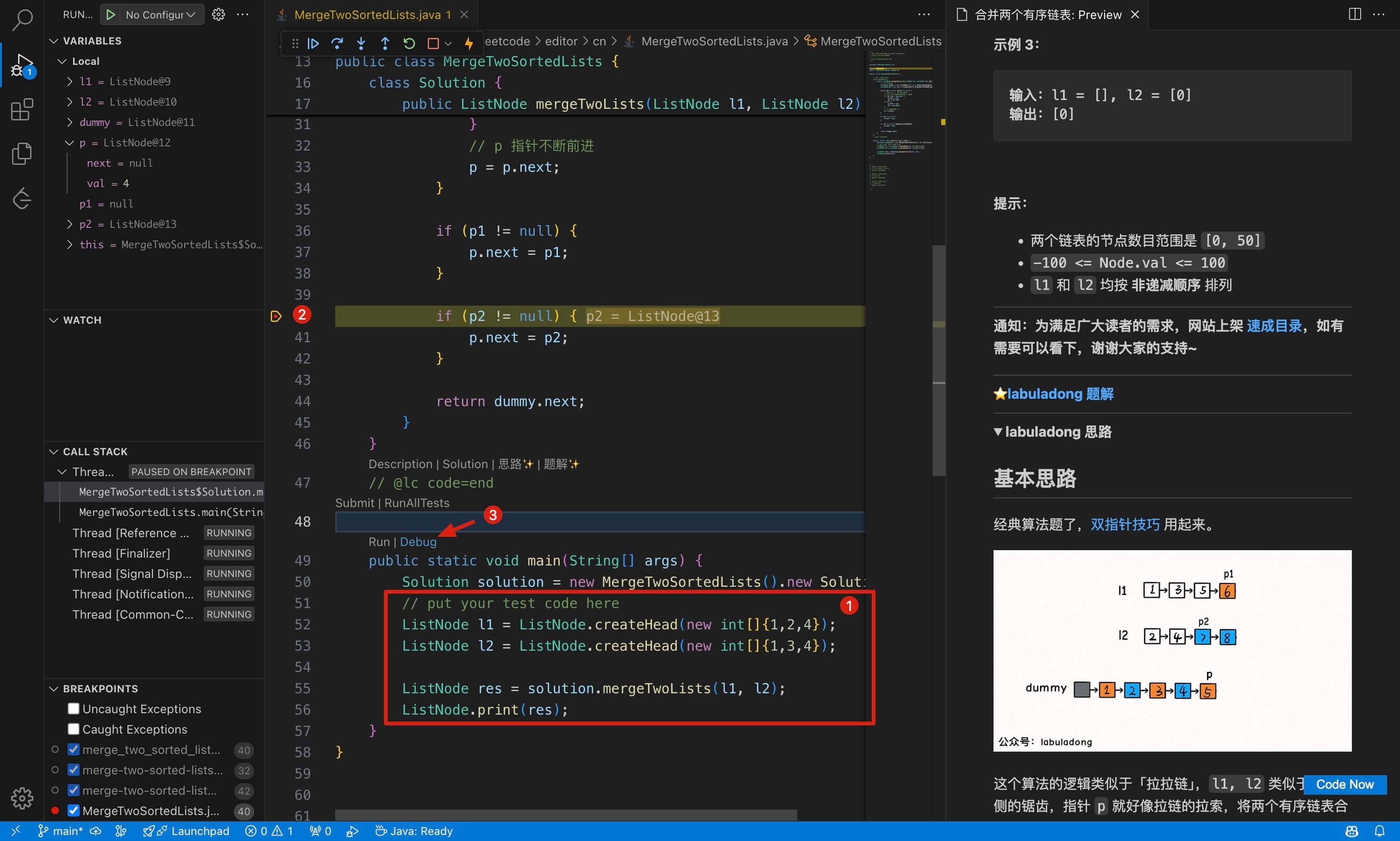
If the Debug
button does not appear above your main
function, it might be because the Extension Pack for Java is not installed. Please install it and try again.
Python Configuration Method
The configuration for Python code templates is as follows:
import sys
import os
sys.path.append(os.path.join(os.path.dirname(__file__), '..'))
from typing import *
from common.node import *
${question.code}
if __name__ == '__main__':
solution = Solution()
\# your test code here
After configuration, open the LeetCode problem to write algorithm code, set breakpoints, and prepare test inputs. Note the run icon at the top right of the file; there is a Debug Python File
option in the dropdown. Click it to debug the code step by step:
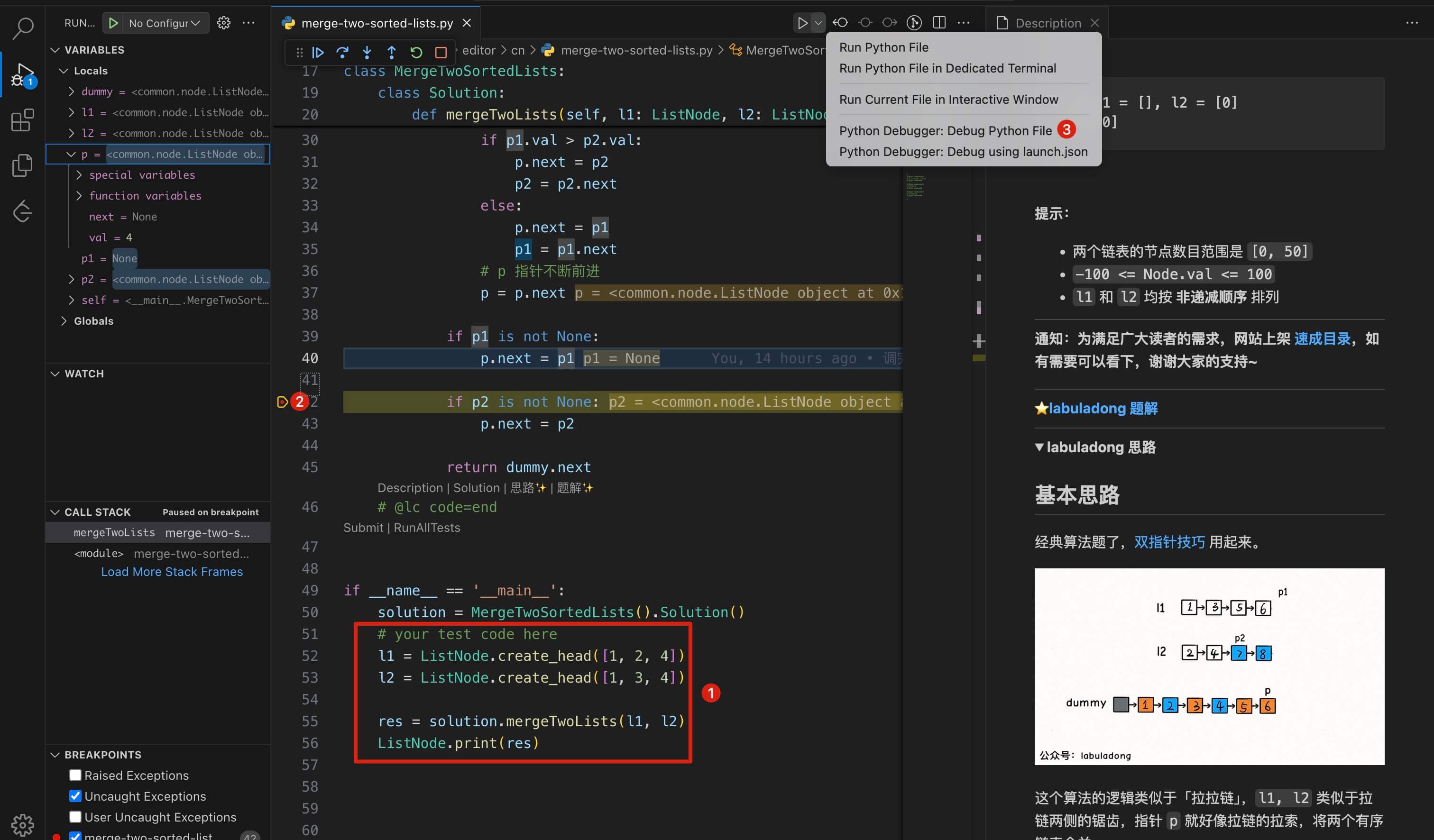
If you cannot see the Debug Python File
option, it may be because the Python Debugger plugin is not installed. Please install it and try again.
C++ Configuration Method
The code template configuration for C++ is as follows:
\#include <iostream>
\#include <vector>
\#include <string>
\#include "../common/ListNode.cpp"
\#include "../common/TreeNode.cpp"
using namespace std;
${question.code}
int main() {
Solution solution;
// your test code here
}
After configuration is complete, open the LeetCode problem to write algorithm code, set breakpoints, and prepare your test inputs. Note that there is a CMake
icon in the sidebar, click it and select the build target corresponding to the problem file, then right-click and select Debug
to debug the code line by line:
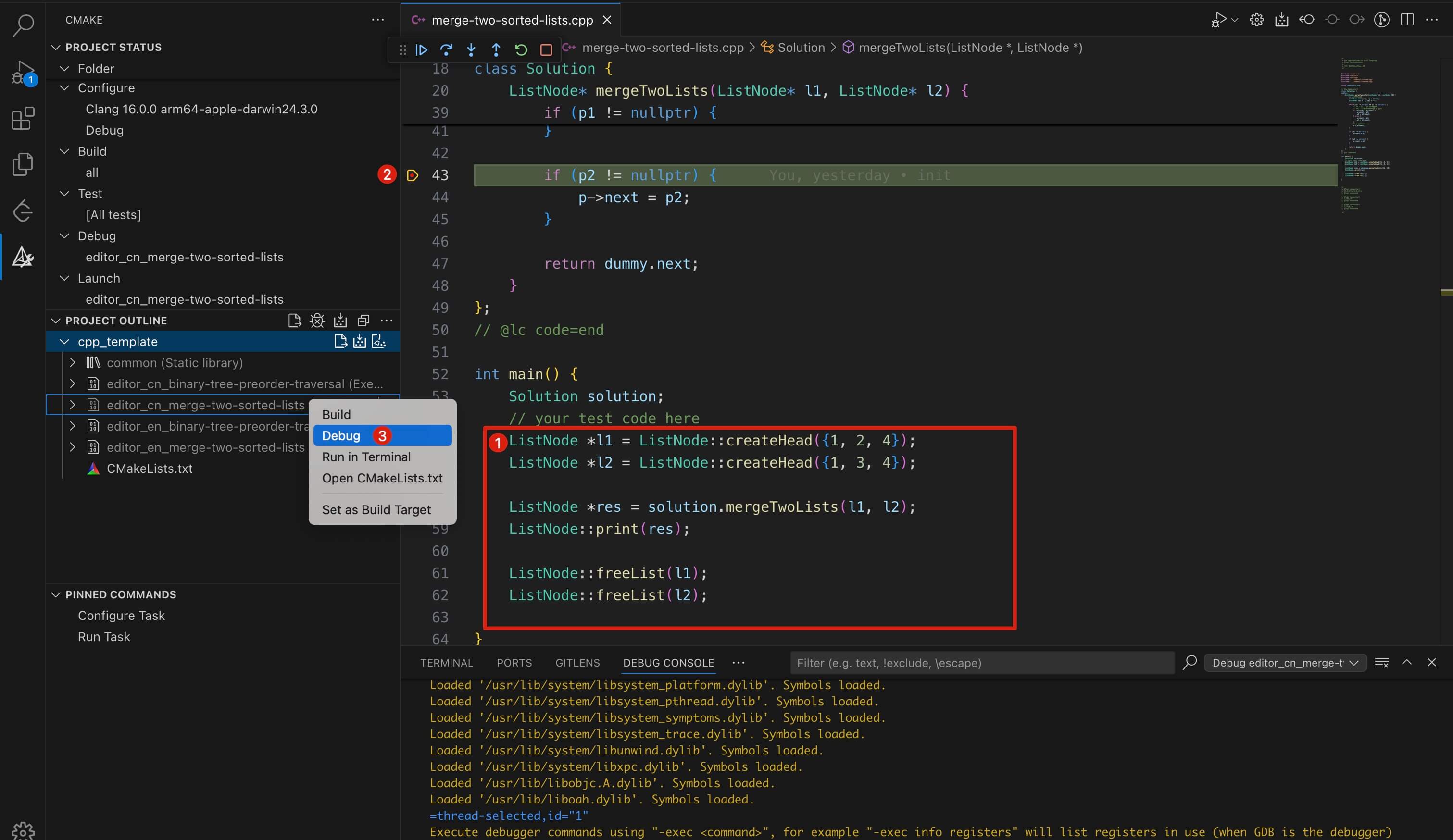
If you do not see the Debug
icon, it may be because the C/C++ Extension Pack plugin is not installed. Please install it and try again.
The C++ project environment configuration can be quite cumbersome. If there is an error running the code, please check:
Your C++ environment should at least be C++ 17 standard, as outdated environments may lack some standard library types, such as
nullopt
.Ensure that your compiler is loading the
CMakeLists.txt
file from thecpp-template
folder, as loading the wrong file will prevent the code from running.
Golang Configuration Method
A Golang package cannot have multiple runnable main functions, but we can use the Golang test file mechanism to bypass this limitation.
The Golang code template configuration is as follows:
package leetcode_solutions
import "testing"
${question.code}
#set($className = $!velocityTool.camelCaseName(${question.titleSlug}))
func Test${className}(t *testing.T) {
// your test code here
}
After configuration, open a LeetCode problem to write algorithm code, set breakpoints, and prepare test inputs. You will see a debug test
button above the TestXXX
function. Click it to debug the code line by line:
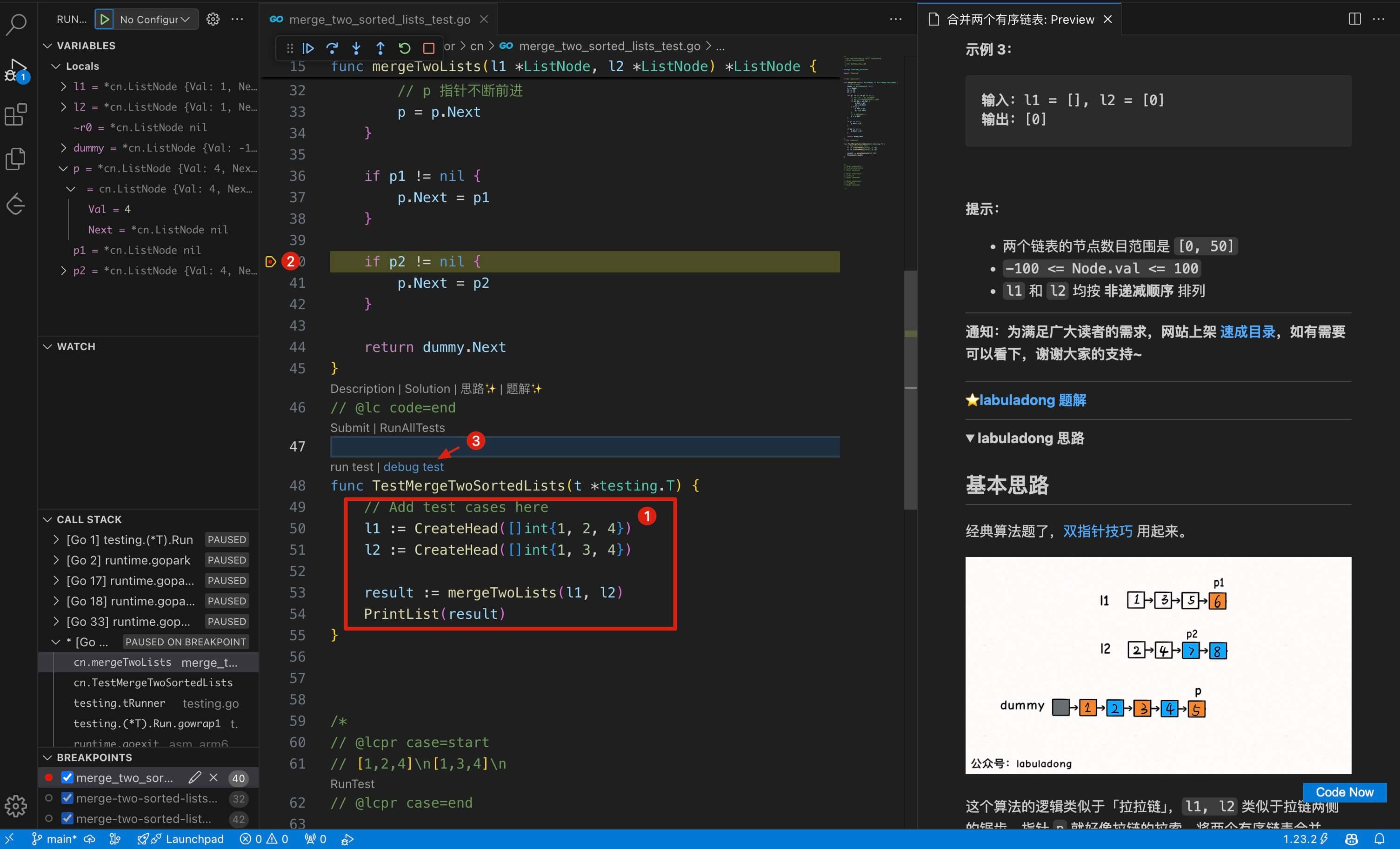
If you do not see the debug test
button, it may be because the Go for Visual Studio Code plugin is not installed. Please install it and try again.
JavaScript Configuration Method
The JavaScript code template configuration is as follows:
import {ListNode} from "../common/listNode.js";
import {TreeNode} from "../common/treeNode.js";
${question.code}
// your test code here
After the configuration is complete, open the LeetCode problem to write your algorithm code, set breakpoints, and prepare the test input. There is a Run and Debug
button on the sidebar, clicking it will allow you to debug the code step by step:
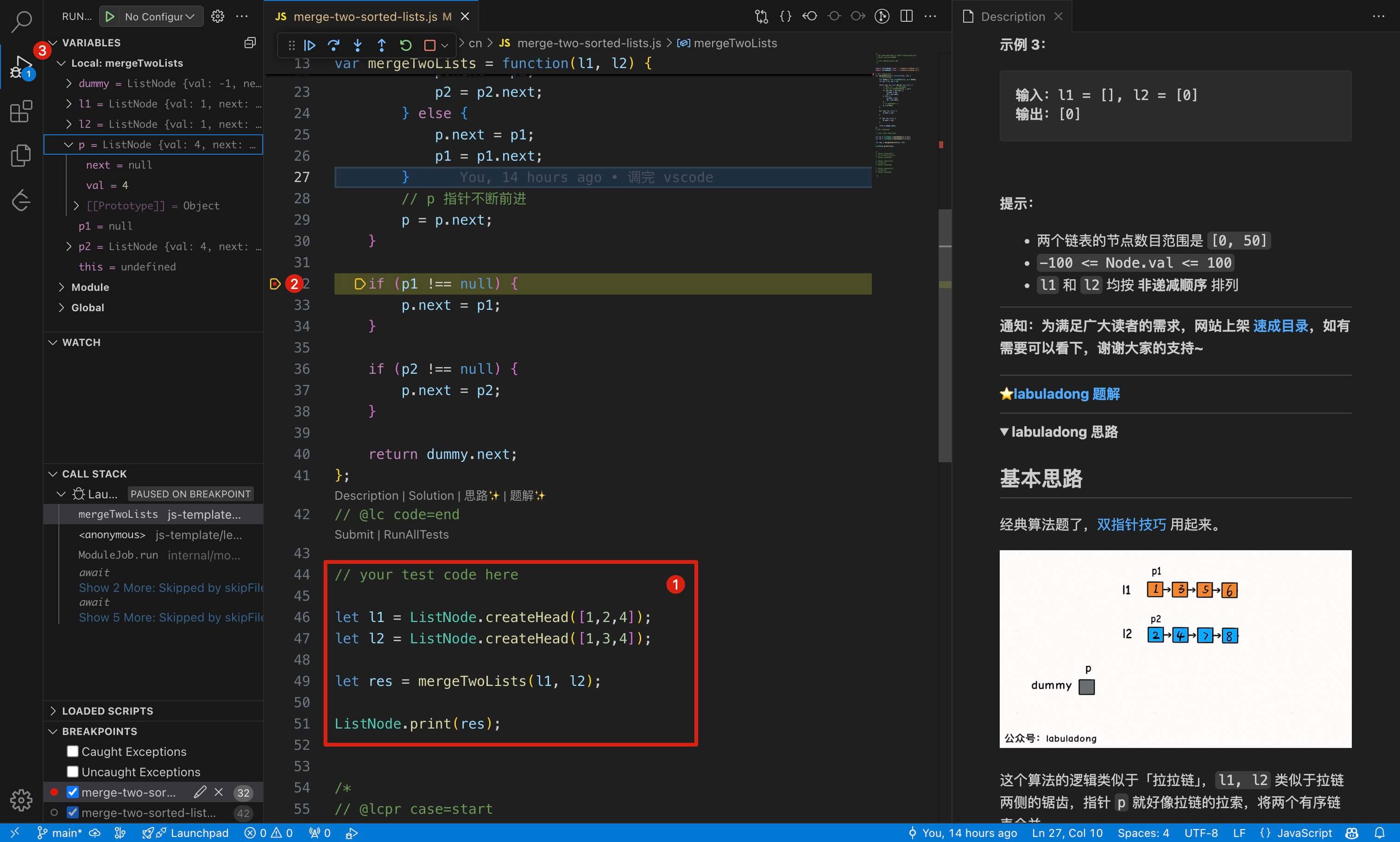
Plugin Configuration
Search for the keyword labuladong
in the settings page of VSCode to see all the configurations related to the plugin, which you can modify according to your needs.
Common configurations:
- Enter the keyword
labuladong default language
to set the programming language for problem solving. - Enter the keyword
labuladong show locked
to configure whether to display the LeetCode plus problems.
Update Method
By default, VSCode will automatically detect and update the plugin.
Changelog
Common Issue Solutions
Error command xxx not found
?
This error indicates that the plugin hasn't loaded yet. Please click the LeetCode icon in the VSCode sidebar to start and load the plugin.
Unable to Log into LeetCode?
Ensure you have copied the cURL for login as per the above method; ensure you have selected the correct login platform; ensure you are already logged into the LeetCode account on the web.
If it displays login...
and doesn't show a successful login, it is likely a network issue. Try closing all network proxies or changing networks.
No ✨ Mark and Solution/Idea Button?
If you don't see the solution/idea button, it is likely because the plugin data failed to load. You can manually trigger data loading as follows:
- Press the shortcut key
F1
to open the VSCode plugin command input box. - Enter
labuladong
in the input box to find aRefresh labuladong.onlin data
command. - Click this command to manually trigger data loading. Once completed, the problem list should display the ✨ mark, and the corresponding solution/idea button will appear.
If no window pops up after the operation, or data loading is slow, try closing all network proxies or changing networks.
No Response to Manual Data Refresh?
This is usually a network issue. Try closing all network proxies or changing networks.
Setting the Name and Path of Code Files?
The plugin supports setting the storage name of code files according to different programming languages.
Search the configuration keyword labuladong-leetcode filepath
in the VSCode settings page to see an Edit in setting.json
option. Click it to write your required configuration into the settings.json
file.
For example, you can set the naming convention for Python3 code files as follows:
"labuladong-leetcode.filePath": {
"python3": {
"filename": "${id}.${cn_name}.${ext}"
},
// ...
}
Available variables are:
Problem ID | Problem Name | Problem Chinese Name | Extension | Current Date | Camel Case Name | Snake Case Name | Kebab Case Name |
---|---|---|---|---|---|---|---|
$ | $ | $ | $ | $ | $ | $ | $ |
Bug Report
You can create an issue on GitHub to report problems: