Labuladong Algo Notes
Overview of Features
Rapid Mastery
Plugin Suite
Algorithm Visualization Panel
Code Image Annotations
Support for Major Programming Languages
Discussion Section
Reading History
Algorithm Visualization
Interactive algorithm visualization panels. Every problem solution has a corresponding visualization panel.
Algorithm Execution Flow Visualization The left side contains the algorithm code, and the right side contains the visualization panel, both of which are interactive to help you understand.
Data Structure Visualization Includes data structures such as linked lists, arrays, hash tables, binary trees, multi-way trees, binary heaps, etc.
Recursive Algorithm Visualization By combining the "framework thinking" approach, the exhaustive tree of BFS/DFS algorithms is visualized, helping you understand recursive algorithms from a tree perspective.
This feature is supported across the website and all related plugins
Algo Visualization Example
Algorithm Game
The website comes with several games, requiring players to use algorithms to complete game tasks or develop game functions, making it more interesting.
华容道游戏
Image Annotations
For more complex algorithms, the code contains lightbulb icons. Hovering over them will display a corresponding image to assist your understanding.
This feature is supported across the website and all related plugins
class Solution {
public ListNode detectCycle(ListNode head) {
ListNode fast, slow;
fast = slow = head;
while (fast != null && fast.next != null) {
fast = fast.next.next;
slow = slow.next;
if (fast == slow) break;
}
// 上面的代码类似 hasCycle 函数
if (fast == null || fast.next == null) {
// fast 遇到空指针说明没有环
return null;
}
// 重新指向头结点
slow = head;
// 快慢指针同步前进,相交点就是环起点
while (slow != fast) {
fast = fast.next;
slow = slow.next;
}
return slow;
}
}
class Solution {
public:
ListNode *detectCycle(ListNode *head) {
ListNode *fast, *slow;
fast = slow = head;
while (fast != nullptr && fast->next != nullptr) {
fast = fast->next->next;
slow = slow->next;
if (fast == slow) break;
}
// 上面的代码类似 hasCycle 函数
if (fast == nullptr || fast->next == nullptr) {
// fast 遇到空指针说明没有环
return nullptr;
}
// 重新指向头结点
slow = head;
// 快慢指针同步前进,相交点就是环起点
while (slow != fast) {
fast = fast->next;
slow = slow->next;
}
return slow;
}
};
class Solution:
def detectCycle(self, head: ListNode):
fast, slow = head, head
while fast and fast.next:
fast = fast.next.next
slow = slow.next
if fast == slow:
break
# 上面的代码类似 hasCycle 函数
if not fast or not fast.next:
# fast 遇到空指针说明没有环
return None
# 重新指向头结点
slow = head
# 快慢指针同步前进,相交点就是环起点
while slow != fast:
fast = fast.next
slow = slow.next
return slow
func detectCycle(head *ListNode) *ListNode {
fast, slow := head, head
for fast != nil && fast.Next != nil {
fast = fast.Next.Next
slow = slow.Next
if fast == slow {
break
}
}
if fast == nil || fast.Next == nil {
return nil
}
slow = head
for slow != fast {
fast = fast.Next
slow = slow.Next
}
return slow
}
var detectCycle = function(head) {
let fast, slow;
fast = slow = head;
while (fast !== null && fast.next !== null) {
fast = fast.next.next;
slow = slow.next;
if (fast == slow) break;
}
// 上面的代码类似 hasCycle 函数
if (fast === null || fast.next === null) {
// fast 遇到空指针说明没有环
return null;
}
// 重新指向头结点
slow = head;
// 快慢指针同步前进,相交点就是环起点
while (slow !== fast) {
fast = fast.next;
slow = slow.next;
}
return slow;
};
Chrome Plugin for LeetCode
LeetCode Web Enhancements Marks problems I’ve covered on the problem list; adds "Solution" and "Analysis" buttons to the problem details page, allowing you to view my solution or visit the site for detailed explanations.
Handy Features Code image annotations, support for all major programming languages, and more.
Algorithm Visualization Almost all problem "Solution" pop-ups include animations to help you better understand algorithms.
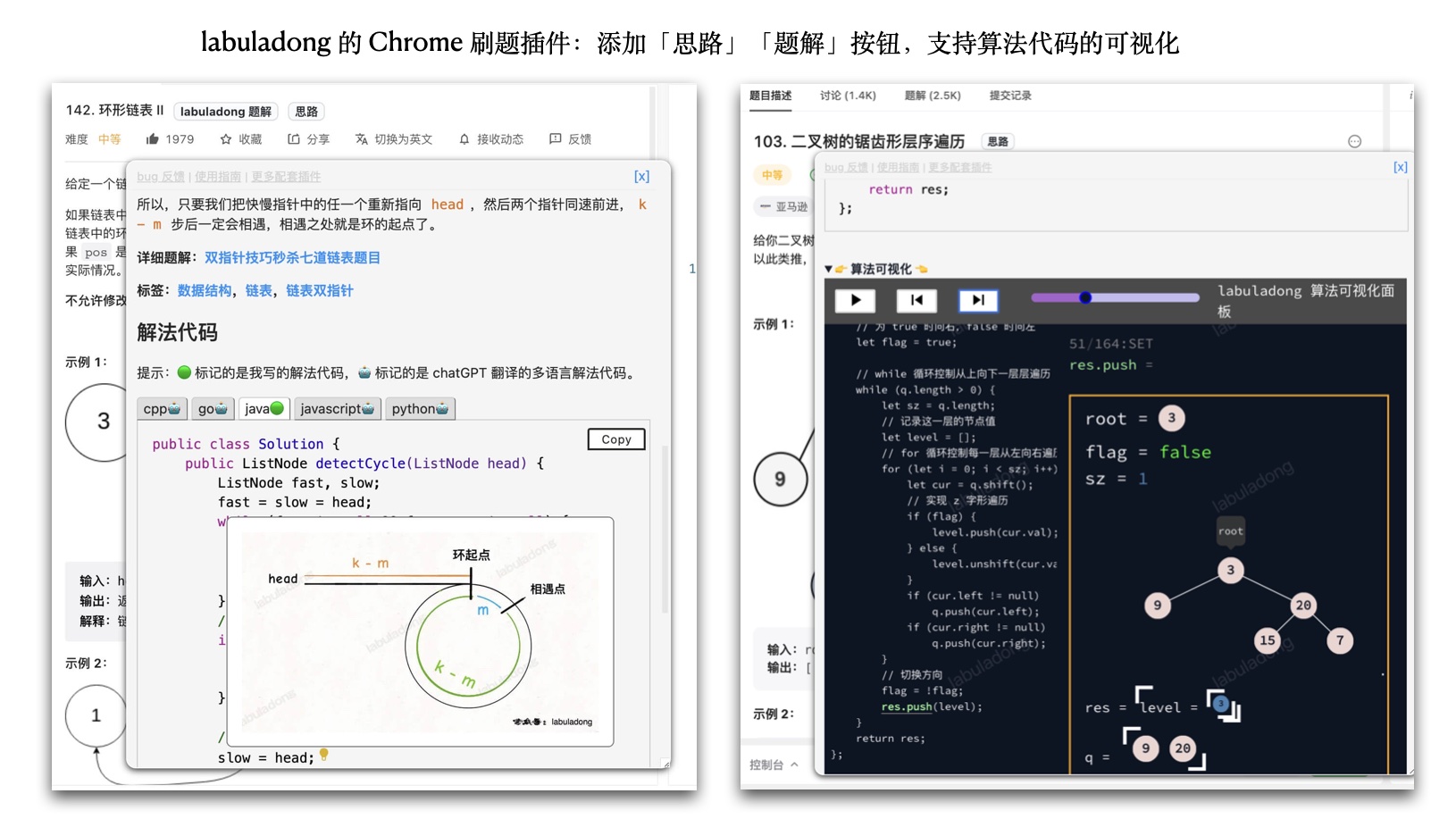
VSCode Plugin for LeetCode
Similar features to the Chrome plugin, for those who prefer not to solve problems via the website.
Solve Problems in VSCode Supports LeetCode problem-solving and integrates my solutions✨ and analyses✨ to facilitate learning.
Handy Features Code image annotations, support for all major programming languages, and configuration of code templates for easy local debugging.
Algorithm Visualization Almost all problem "Solution" pop-ups include animations to help you better understand algorithms.
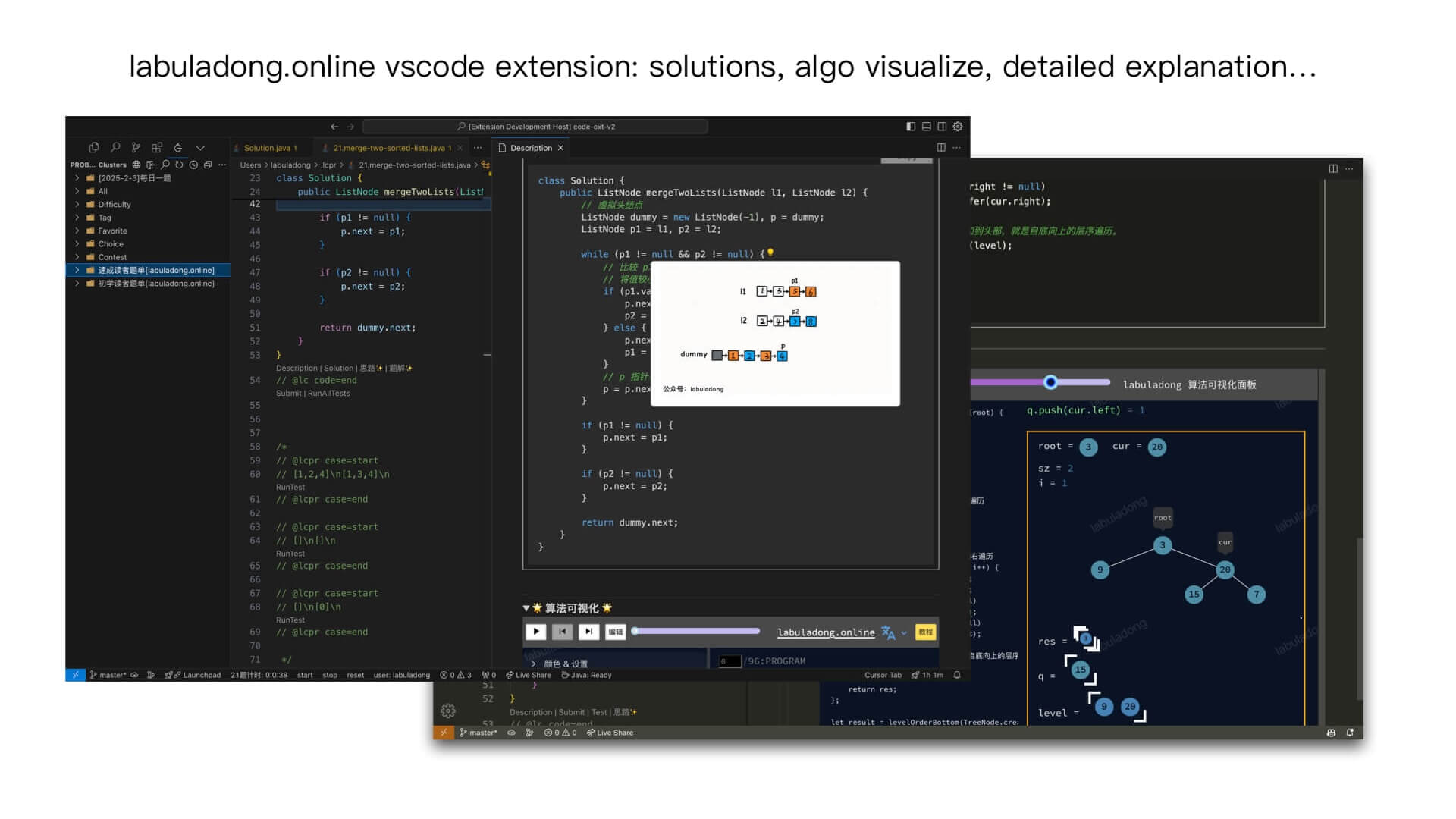
JetBrains Plugin for LeetCode
Similar features to the Chrome plugin, for those who prefer not to solve problems via the website.
Solve Problems in JetBrains IDE Supports solving LeetCode problems and integrates my solutions✨ and analyses✨ for easy learning. Supports the full JetBrains IDE family, including PyCharm, IntelliJ IDEA, and WebStorm.
Handy Features Code image annotations, support for all major programming languages, and configuration of code templates for easy local debugging.
Algorithm Visualization Almost all problem "Solution" pop-ups include animations to help you better understand algorithms.
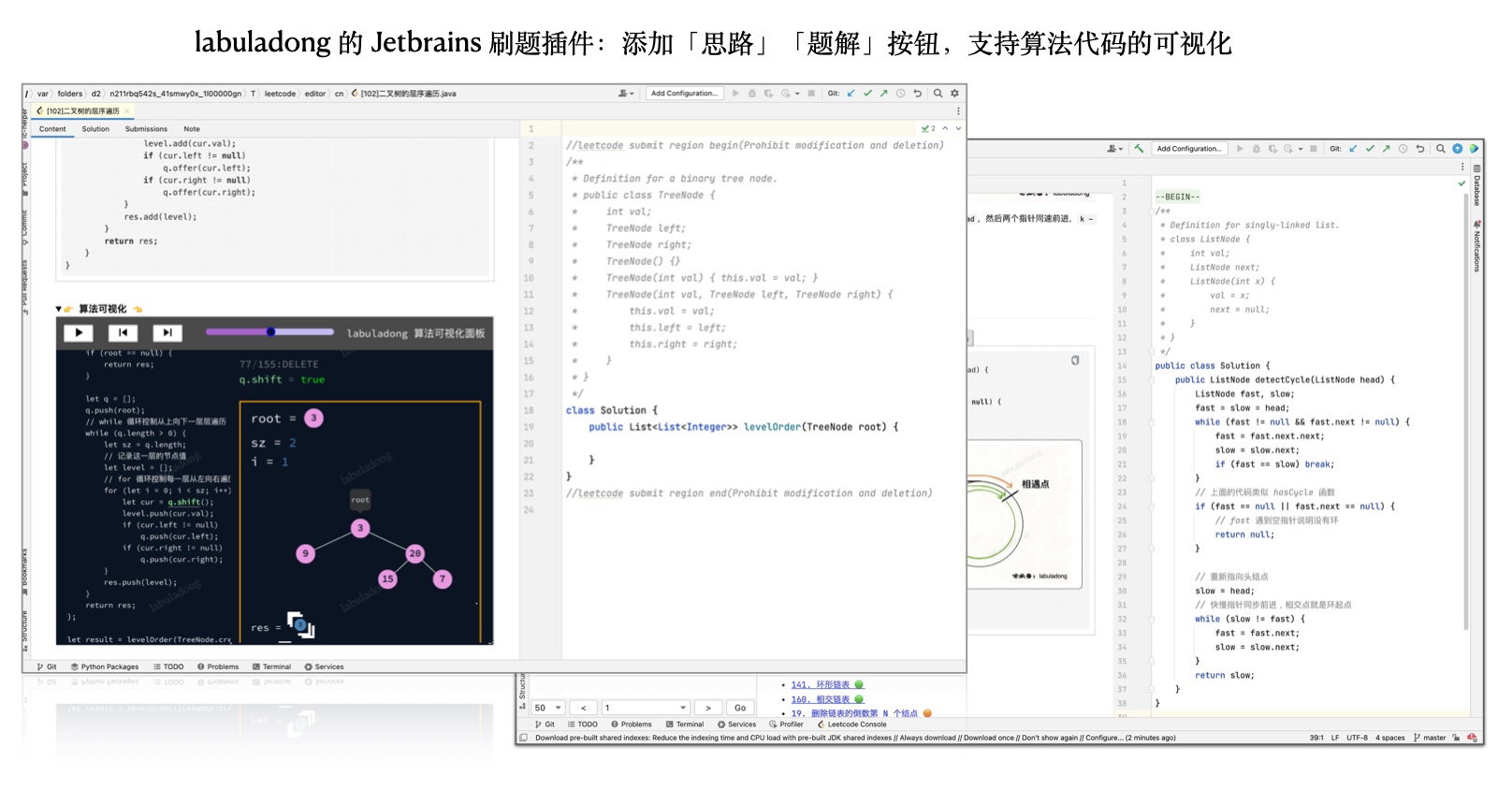