JetBrains Plugin for LeetCode
Relationship Between the Plugin and the Site
The solutions and ideas in the plugin come from the site's articles and exercise explanations. It serves as a supplementary tool for learning and is not mandatory. Please choose based on your needs.
Some readers prefer coding in an editor or IDE rather than on a web page, finding it more convenient for writing and debugging. Therefore, I have developed and maintained plugins across platforms to meet this demand, allowing readers to view the site's problem explanations, visualizations, and problem lists within the plugin.
The JetBrains plugin allows users to solve LeetCode problems in all JetBrains IDEs (such as Intellij, Pycharm, etc.), supporting line-by-line debugging. You can directly view the site's explanation, visualization panels, and access the problem lists from the Quick Mastery Catalog and Beginner's Catalog for easy review:
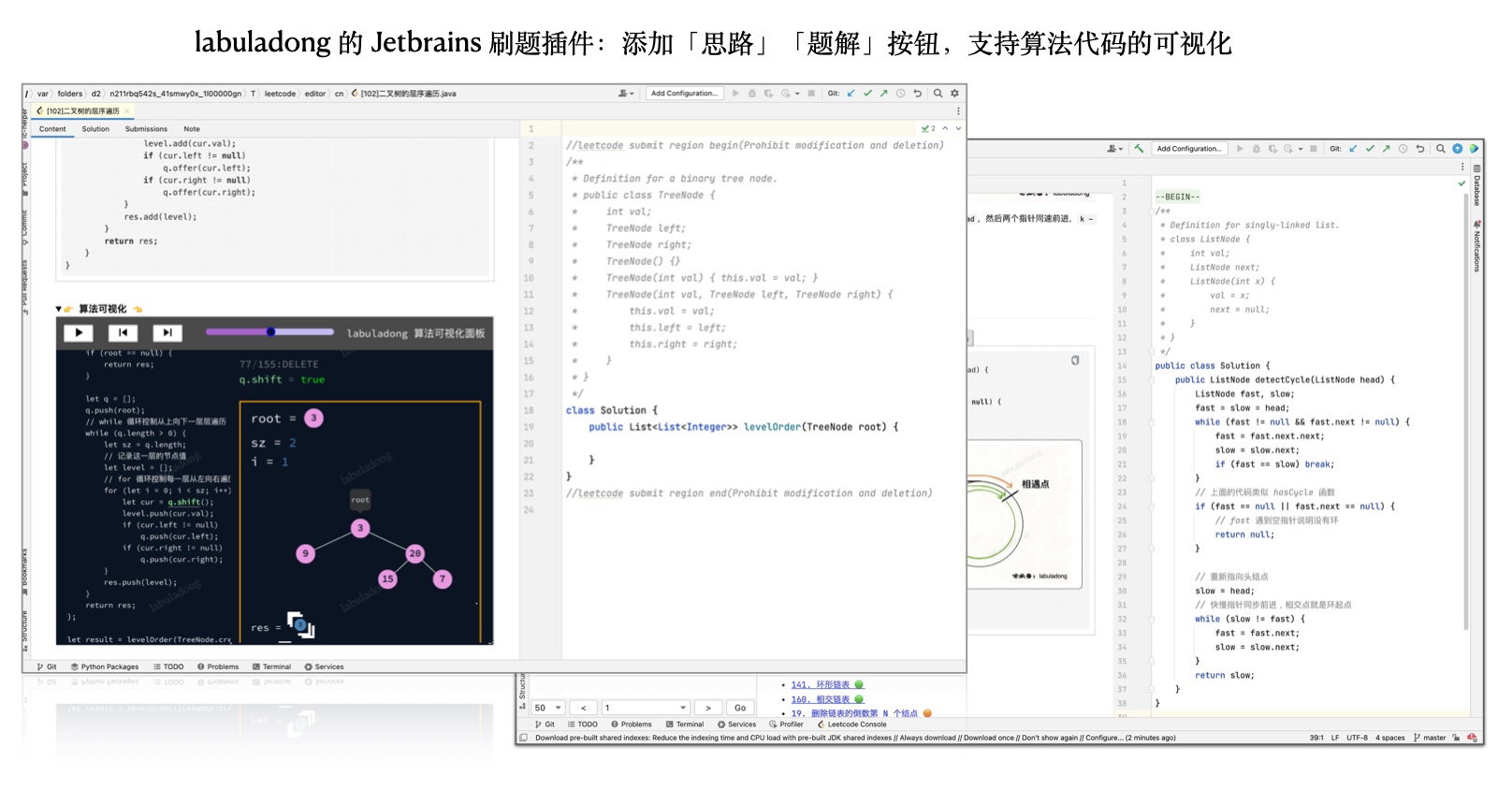
The plugin is developed based on the open-source plugin LeetCode Editor, a tribute to the open-source author @shuzijun!
Installation Method
The full name of my plugin is "LeetCode with labuladong". It can be downloaded by searching for the keyword "labuladong" in the JetBrains series IDE plugin store:
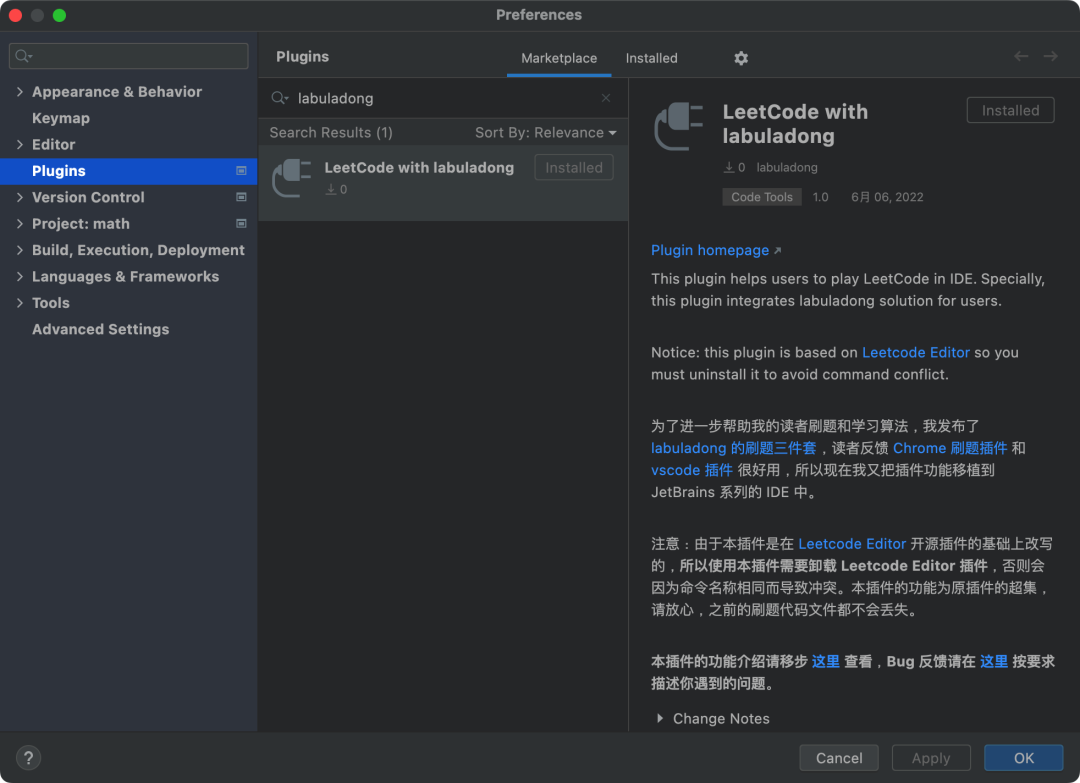
If the plugin cannot be found, it may be a network issue. Installation instructions can be followed on the JetBrains plugin webpage:
https://plugins.jetbrains.com/plugin/19317-leetcode-with-labuladong
Below is an introduction to the basic usage of this plugin and its supplementary features for solving problems.
Feature Display
Upon successful login, problems with solutions or ideas on this site will be marked with ✨. Opening the details page of a problem marked with ✨ will show "labuladong Solution" and "labuladong Idea":
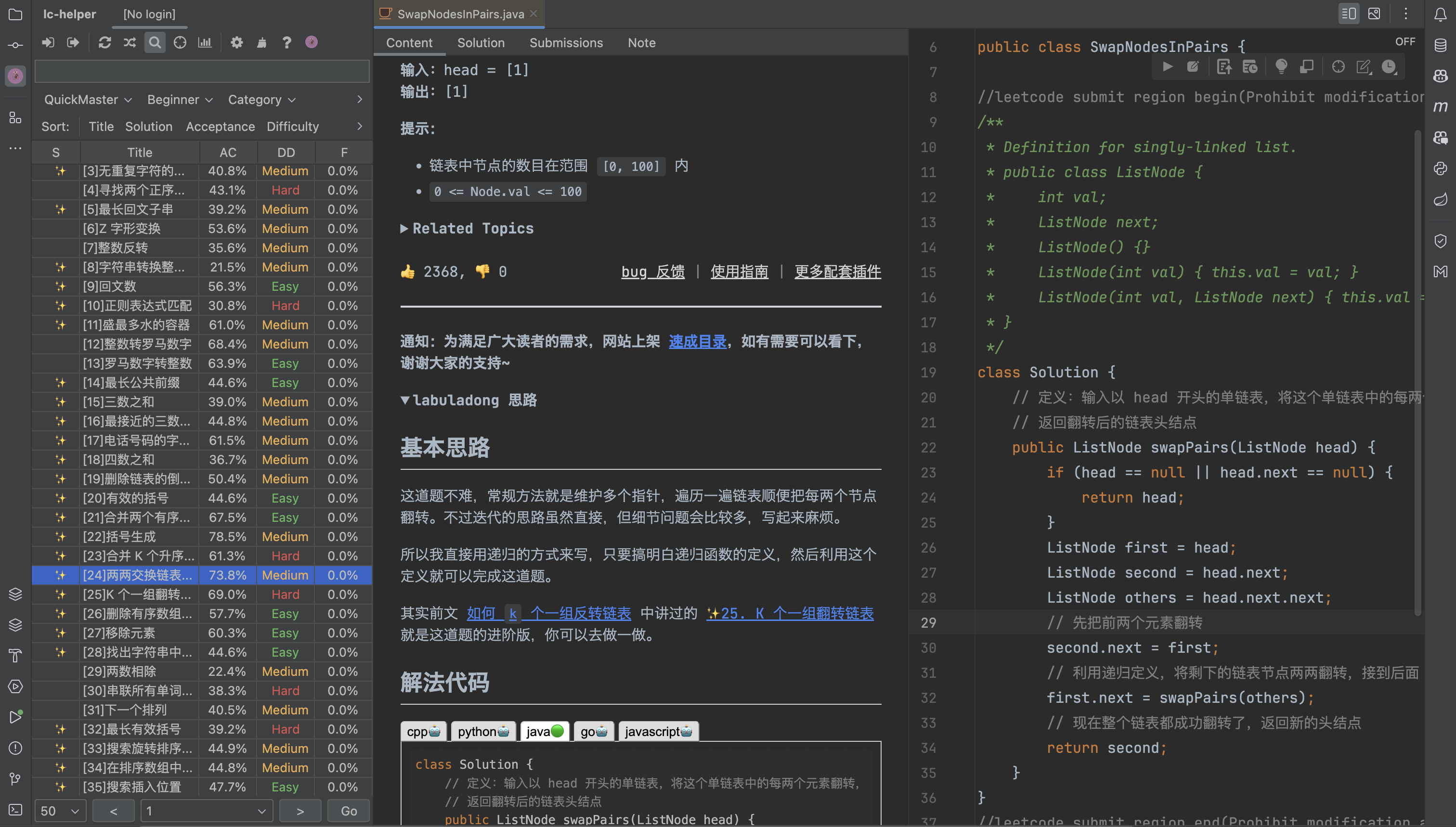
All features of this site are supported by plugins, including image annotations, algorithm visualization, and more:
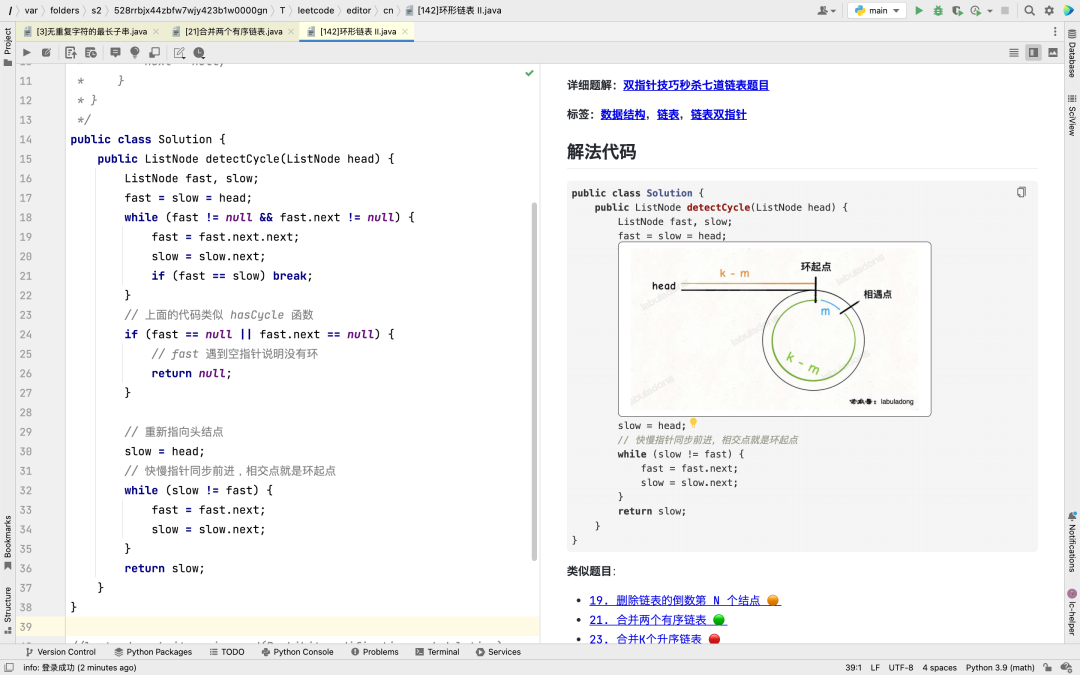
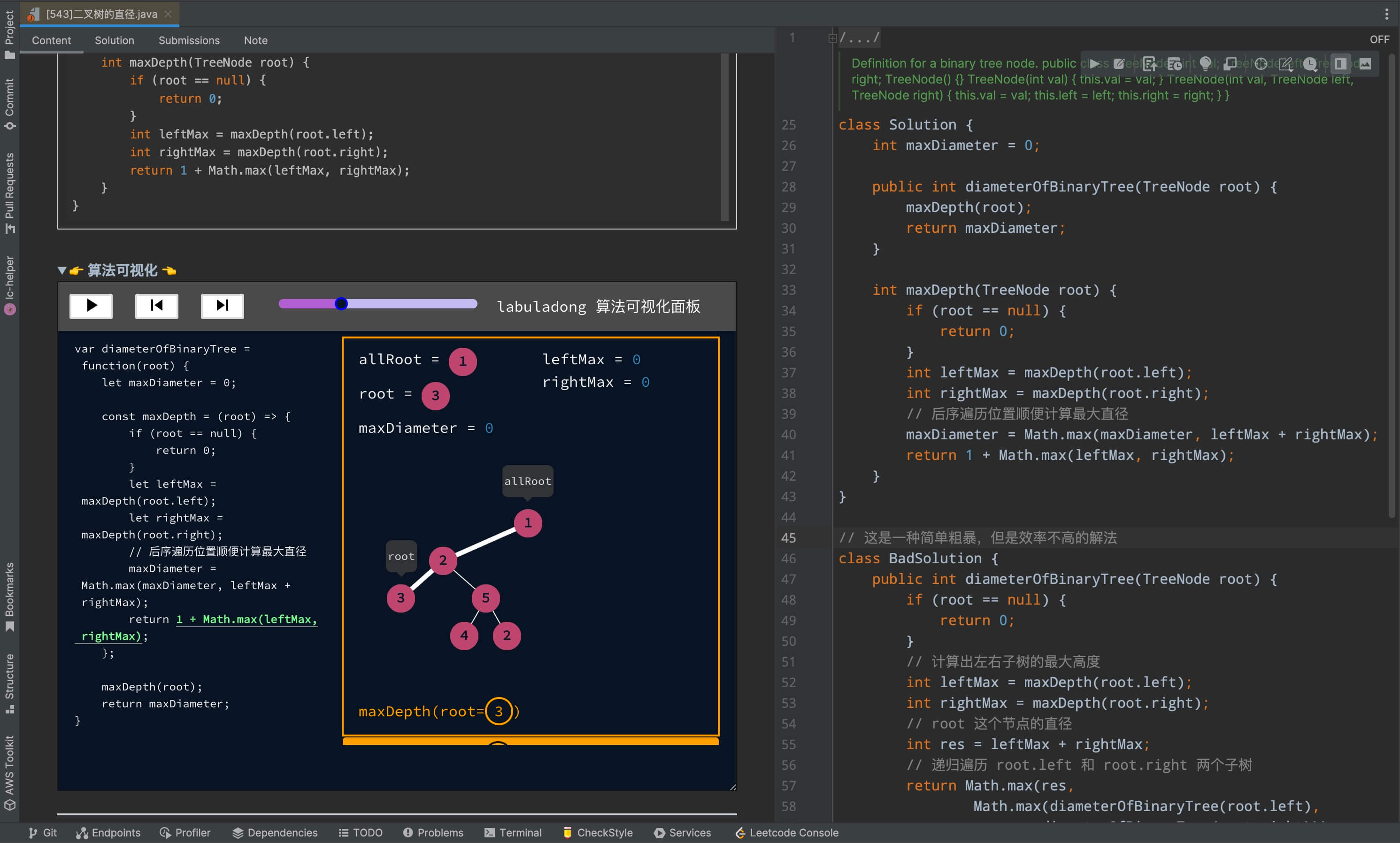
Switching to English for International Users
The default content of the plugin's solutions/ideas/visualization panel is in Chinese. International users can switch to English by changing the Language option to English in the plugin's settings page.
Login to LeetCode
After installing the plugin, an icon will appear in the sidebar. Click the settings button and configure as shown below:
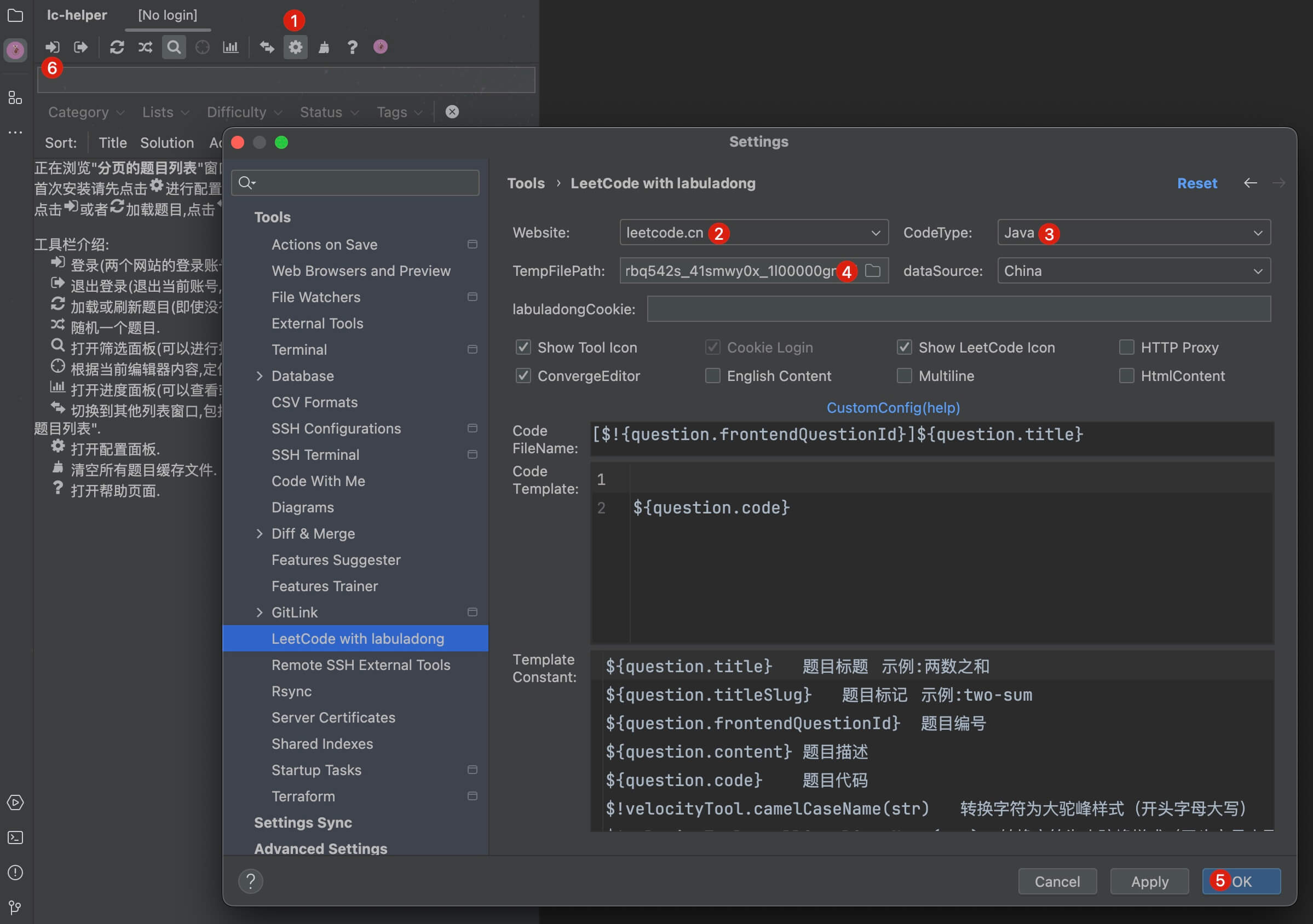
1️⃣ Click the settings button to enter the settings page.
2️⃣ Choose the site to log in to, either the English version LeetCode or the Chinese version 力扣.
3️⃣ Set the programming language you wish to use.
4️⃣ Set the path to store the code files for the problems.
5️⃣ Click the OK button after completing the settings.
6️⃣ Click the login button.
After clicking the login button, a dialog box will pop up for you to enter a cookie. If you set the login site to the English version of LeetCode, enter the cookie for leetcode.com. If you set it to the Chinese version 力扣, enter the cookie for leetcode.cn:
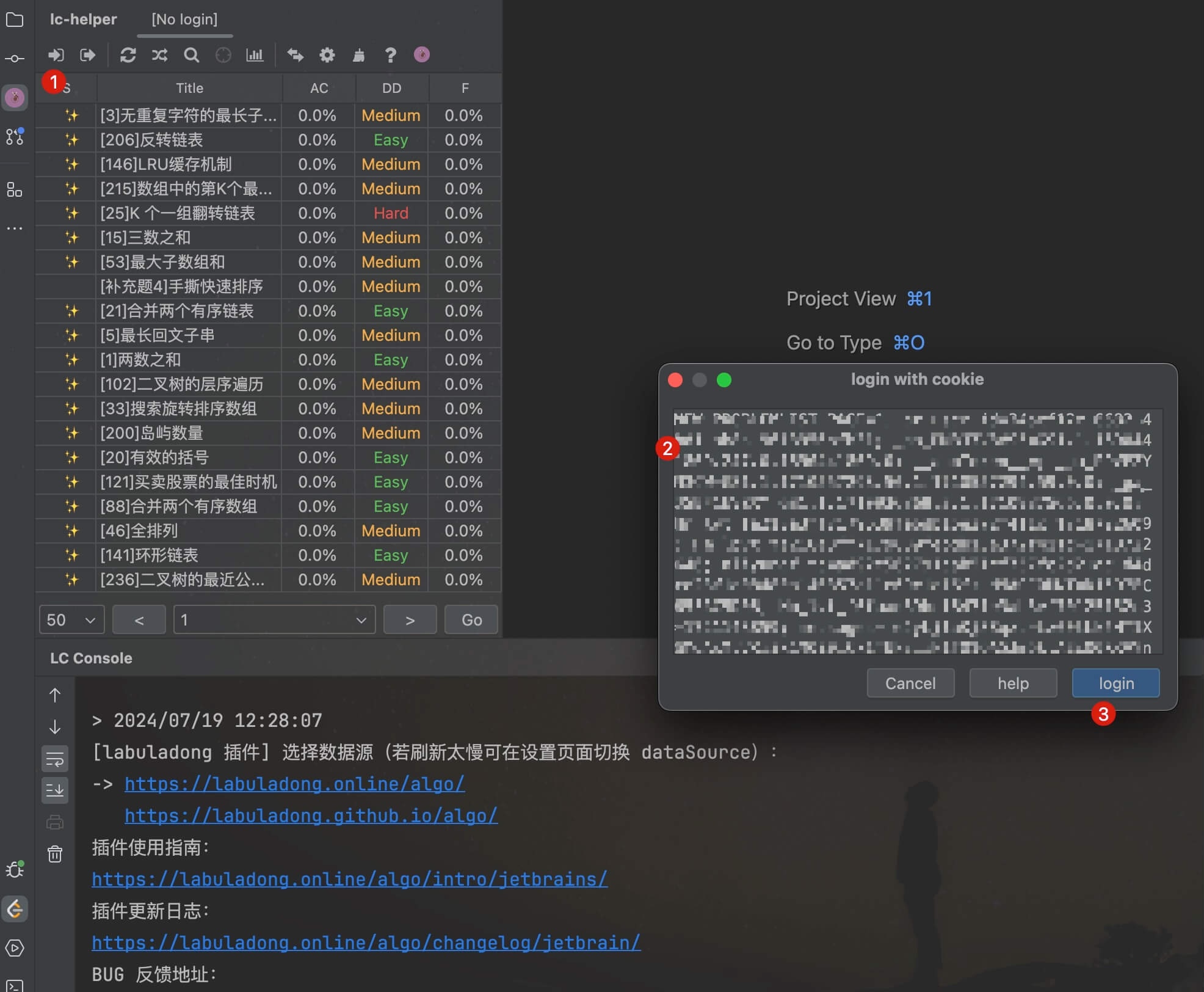
To obtain the cookie for the Chinese 力扣 leetcode.cn, open the official website https://leetcode.cn in your browser, ensuring you are logged into your account. Open the Developer Tools (Chrome can be accessed with F12) and copy the cookie value:
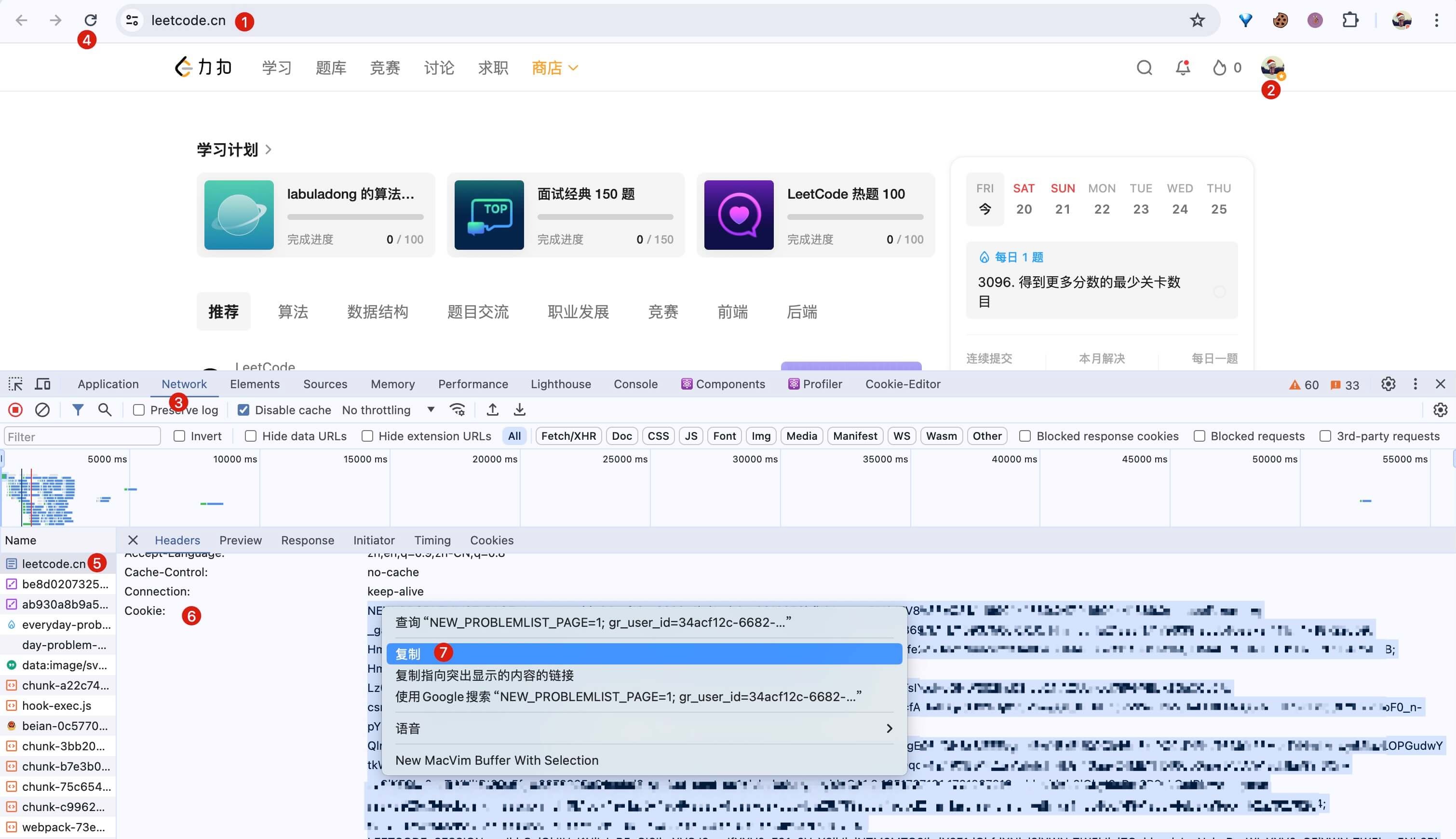
1️⃣ ~ 2️⃣ Open the official website of the Chinese 力扣 https://leetcode.cn and ensure you are logged into your account on the webpage.
3️⃣ Open Developer Tools (Chrome with F12) and click the Network tab.
4️⃣ ~ 6️⃣ Refresh the page, click on the first request, and check its Headers, where there is a Cookie field.
7️⃣ Select the entire cookie string, right-click and copy it.
The method to obtain the cookie for the English LeetCode is similar. Simply open the English version LeetCode website https://leetcode.com and repeat the above steps. It will not be repeated here.
Paste the cookie into the plugin and click login. You should be able to successfully log into your 力扣/LeetCode account in the plugin and start solving problems.
Local Debugging of Algorithm Code
The algorithm code for each problem is essentially an ordinary code file, and theoretically can be debugged and run locally, but there are a few issues:
LeetCode has some built-in types, such as
ListNode, TreeNode
, and the test case inputs for the problems are in array form. You need to implement these structures yourself and convert the input data of the problem.Additional configuration is needed for the features of different programming languages to allow the code editor to correctly recognize the project structure. Otherwise, you may encounter issues such as being unable to run the code locally or use auto-completion.
To address these issues, I have developed a solution. By following the steps below to configure the plugin, you can directly run LeetCode problem code locally. Currently, Java/C++/Python/Golang/JavaScript languages are supported.
Step 1: Open the Corresponding Folder in the IDE
I have hosted the configured project template on a Github repository, which you can clone locally:
git clone https://github.com/labuladong/lc-plugin-template.git
# Readers in mainland China can download from Gitee:
git clone https://gitee.com/labuladong/lc-plugin-template.git
If you encounter any issues or have suggestions for improvements while using this, feel free to submit a PR or Issue.
This template contains folders for several programming languages:
- Java folder:
java-template
- C++ folder:
cpp-template
- Python folder:
python-template
- Golang folder:
go-template
- JavaScript folder:
js-template
Open the corresponding folder in the IDE according to your programming language.
Step 2: Configure for Different Languages
Click the gear icon to open the plugin configuration page. There are several configuration options that need to be set according to the programming language you use for coding:
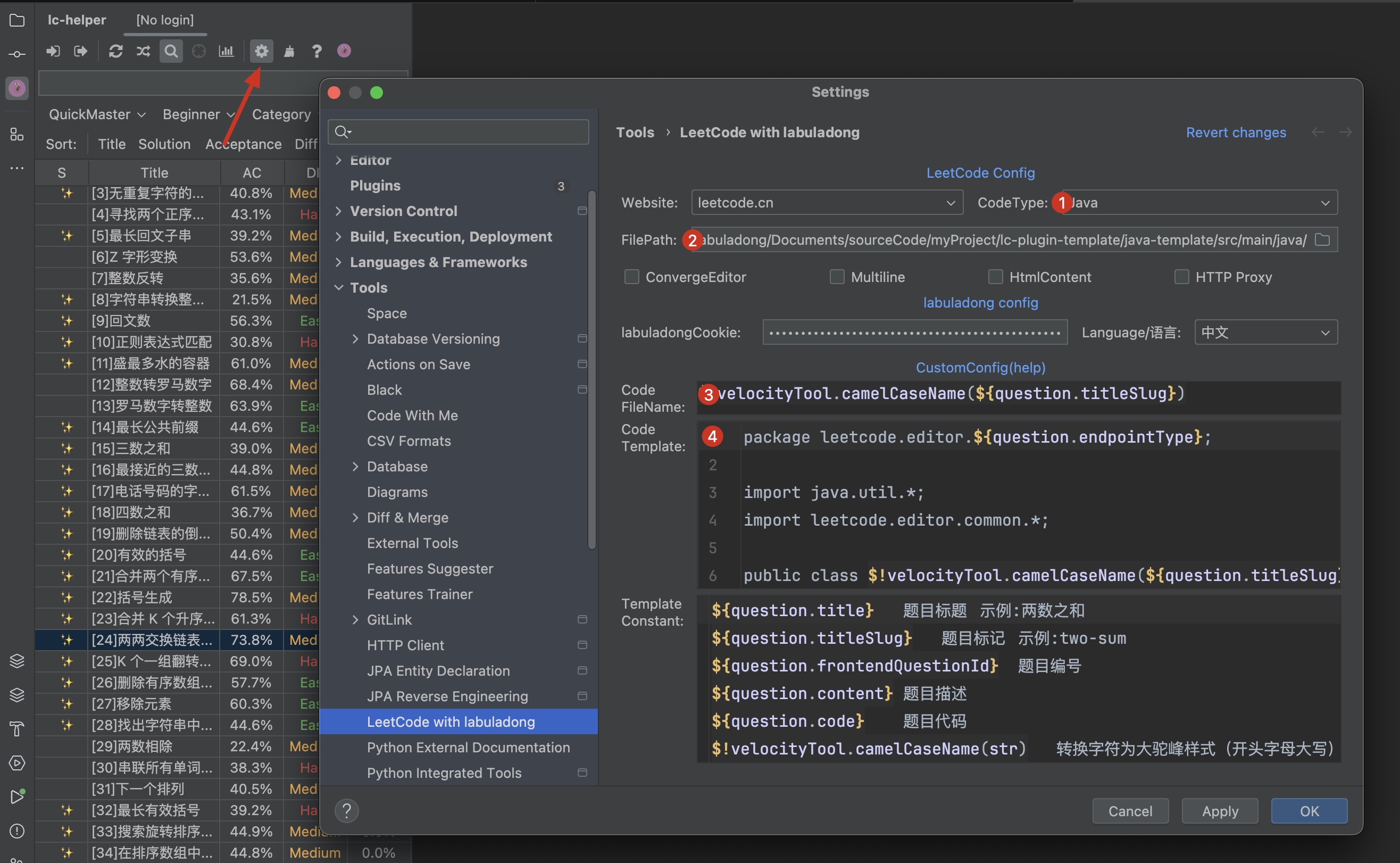
CodeType
: The programming language you are using.FilePath
: The path where the code file is stored.Code Filename
: The name of the code file.CodeTemplate
: The code template, which can automatically add package names,main
function, etc.
Below is a description for each language. Use /<your>/<path>/<to>/lc-plugin-template/
to represent the path where the lc-plugin-template
folder is cloned locally.
Tip
On Windows, the path separator is \
instead of /
, and the path to the lc-plugin-template
folder would look like this:
C:\<your>\<path>\<to>\lc-plugin-template\
Please modify according to actual circumstances.
Java
Jetbrains IDE:Intellij IDEA
CodeType:Java
FilePath:
/<your>/<path>/<to>/lc-plugin-template/java-template/src/main/java
CodeFilename:
$!velocityTool.camelCaseName(${question.titleSlug})
CodeTemplate:
package leetcode.editor.${question.endpointType};
import java.util.*;
import leetcode.editor.common.*;
public class $!velocityTool.camelCaseName(${question.titleSlug}) {
${question.codeWithIndent(4)}
public static void main(String[] args) {
Solution solution = new $!velocityTool.camelCaseName(${question.titleSlug})().new Solution();
// put your test code here
}
}
After the configuration is complete, you can open the problem to write a solution, then add test code and set breakpoints in the main
function to debug the Java algorithm:
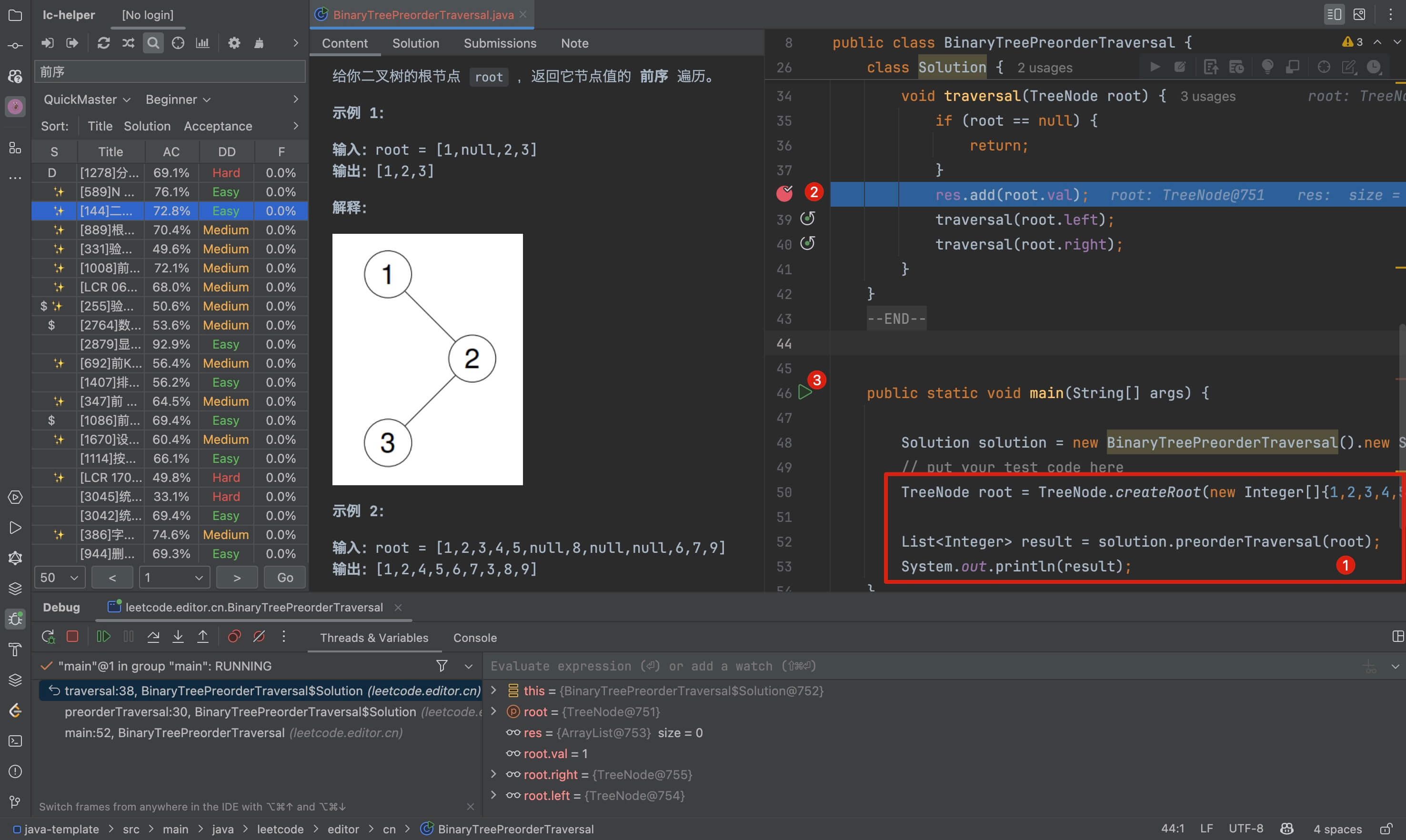
C++
IDE:CLion
CodeType:C++
FilePath:
/<your>/<path>/<to>/lc-plugin-template/cpp-template
CodeFilename:
$!velocityTool.camelCaseName(${question.titleSlug})
CodeTemplate:
\#include <iostream>
\#include <vector>
\#include <string>
\#include "../common/ListNode.cpp"
\#include "../common/TreeNode.cpp"
using namespace std;
${question.code}
int main() {
Solution solution;
// your test code here
}
After completing the configuration, you can open the problem to write a solution, then add test code in the main function and set breakpoints to debug the algorithm:
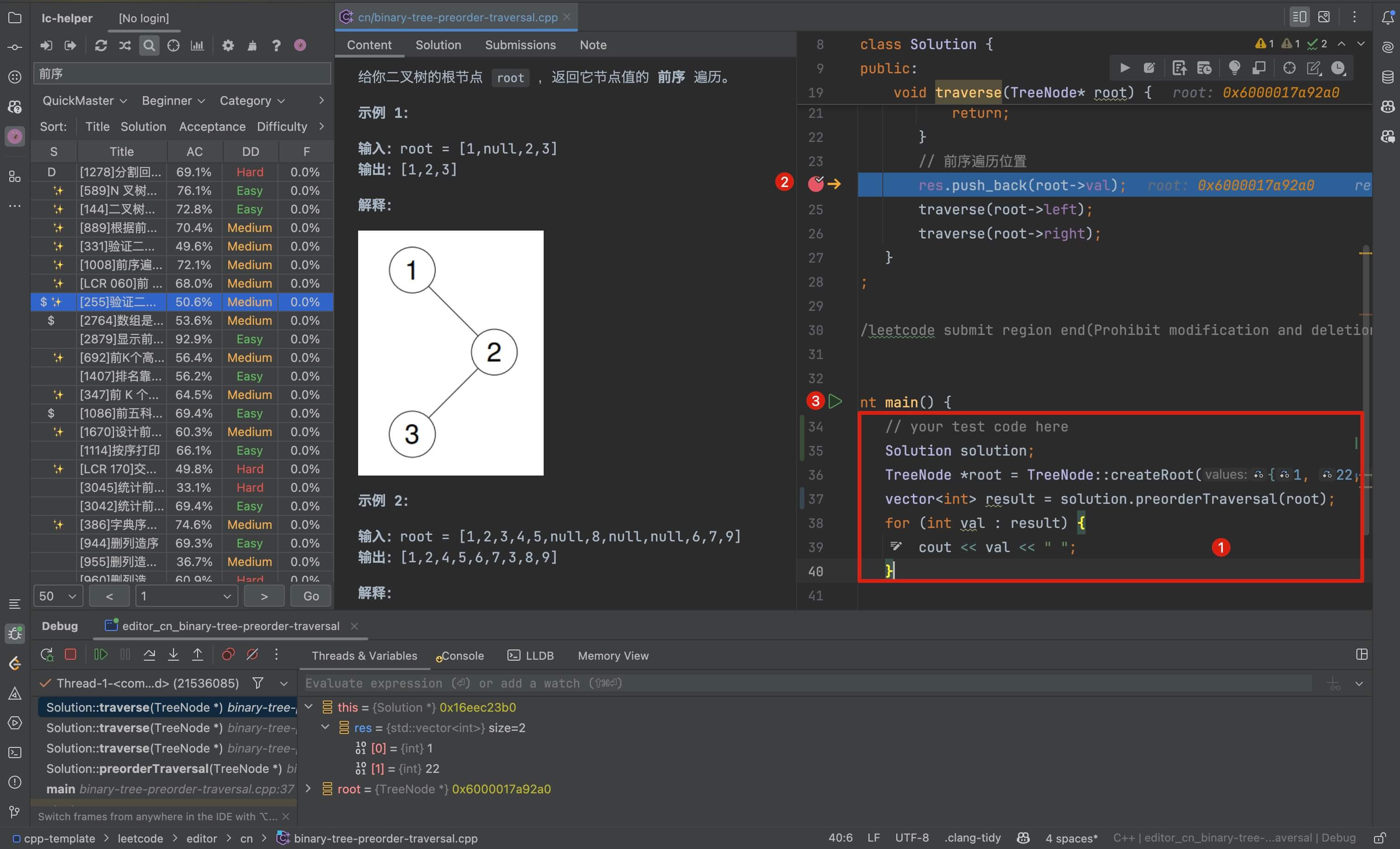
From my tests, when you open a new problem and create a new cpp code file, the IDE does not automatically refresh CMake, which prevents the new code file from compiling. You need to manually refresh CMake here:
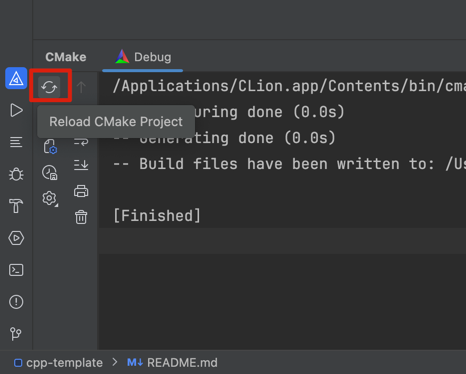
Every time you open a new problem, you need to click this button to manually refresh, which is somewhat inconvenient. We can set a shortcut key to simplify this process.
In Clion's settings page, search for the keyword cmake.reload
to set it up:
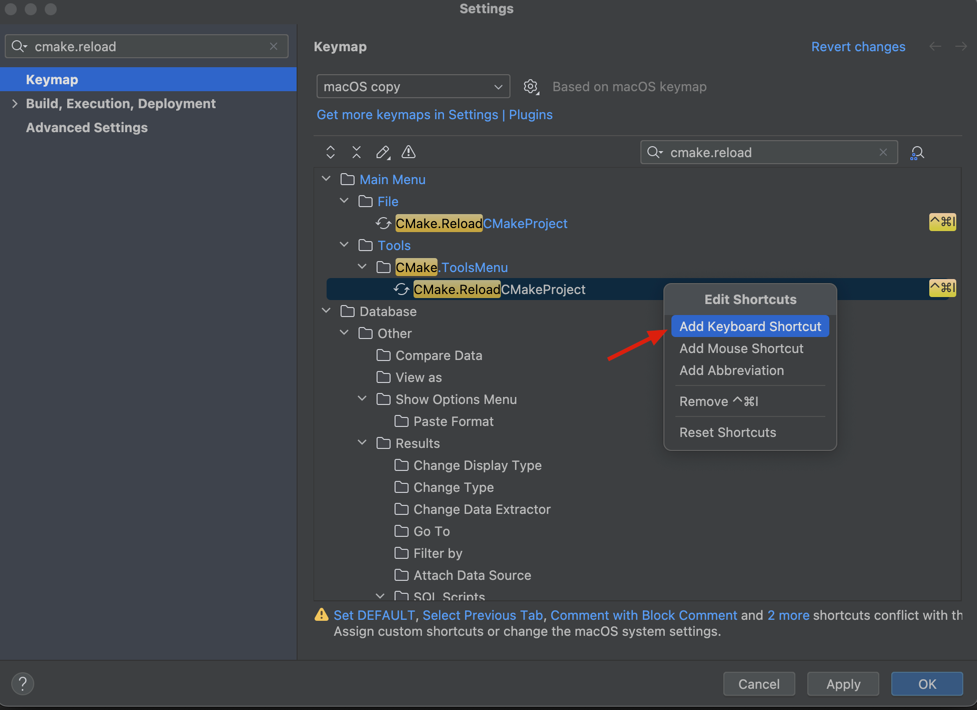
I set the shortcut key to Ctrl + Cmd + I
, but you can set a shortcut key according to your own habits.
In this way, each time you open a new problem, you can quickly reload CMake by pressing the shortcut key, allowing you to debug the newly added code file.
Python
Jetbrains IDE:Pycharm
CodeType:Python3
FilePath:
/<your>/<path>/<to>/lc-plugin-template/python-template
CodeFilename:
$!velocityTool.camelCaseName(${question.titleSlug})
CodeTemplate:
from typing import *
from leetcode.editor.common.node import *
${question.code}
if __name__ == '__main__':
solution = Solution()
# your test code here
After setting up, you can open the problem to write a solution, then add test code and set breakpoints in the main
function to debug Python algorithms:
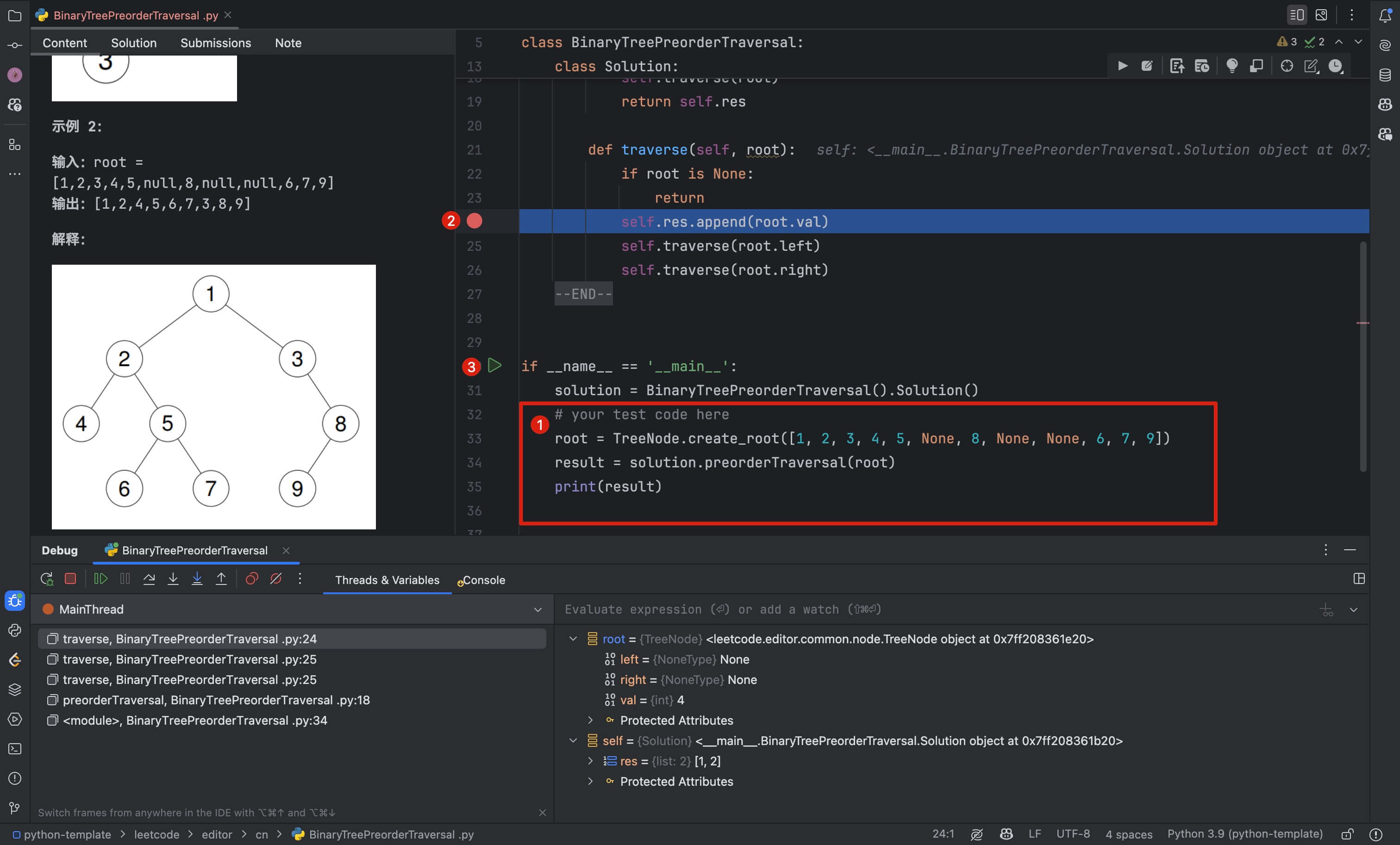
Golang
Jetbrains IDE:Goland
CodeType:Go
FilePath:
/<your>/<path>/<to>/lc-plugin-template/golang-template
CodeFilename:
${question.titleSlug}_test
CodeTemplate:
package leetcode_solutions
import "testing"
${question.code}
func Test$!velocityTool.camelCaseName(${question.titleSlug})(t *testing.T) {
// your test code here
}
Each package in Golang can only have one main function entry point. To bypass this limitation, we utilize the testing file mechanism in Golang, allowing each problem to be individually run and debugged.
After setting up the configuration, you can open the problem to write your solution. Add test code in the TestXXX
function and set breakpoints to debug the algorithm:
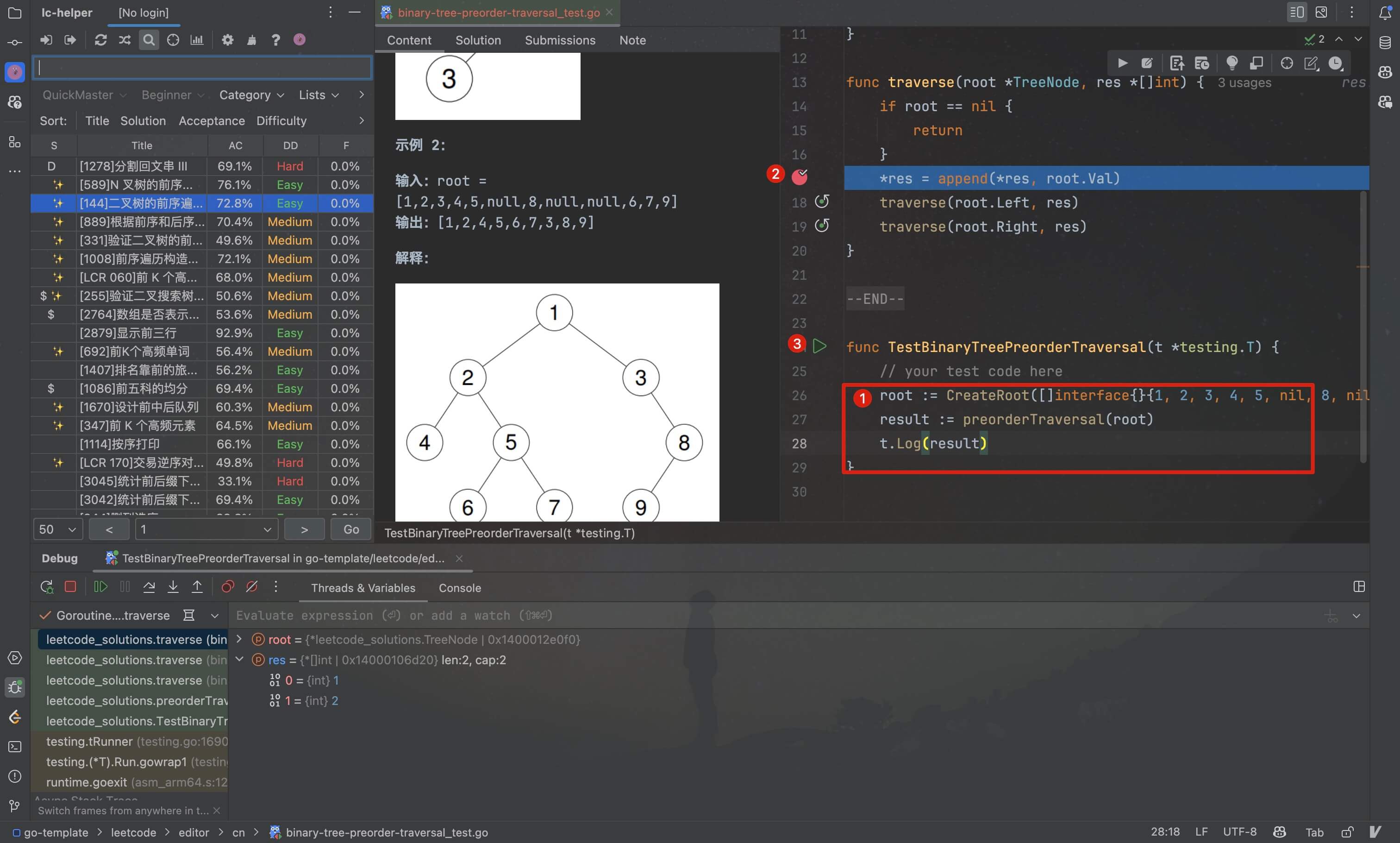
JavaScript
Jetbrains IDE:WebStorm
CodeType:JavaScript
FilePath:
/<your>/<path>/<to>/lc-plugin-template/javascript-template
CodeFilename:
$!velocityTool.camelCaseName(${question.titleSlug})
CodeTemplate:
import {ListNode} from "../common/listNode.js";
import {TreeNode} from "../common/treeNode.js";
${question.code}
// your test code here
After the configuration is complete, you can open the problem to write solutions, add test code and set breakpoints. Click the Debug button in the upper right corner to debug the algorithm:
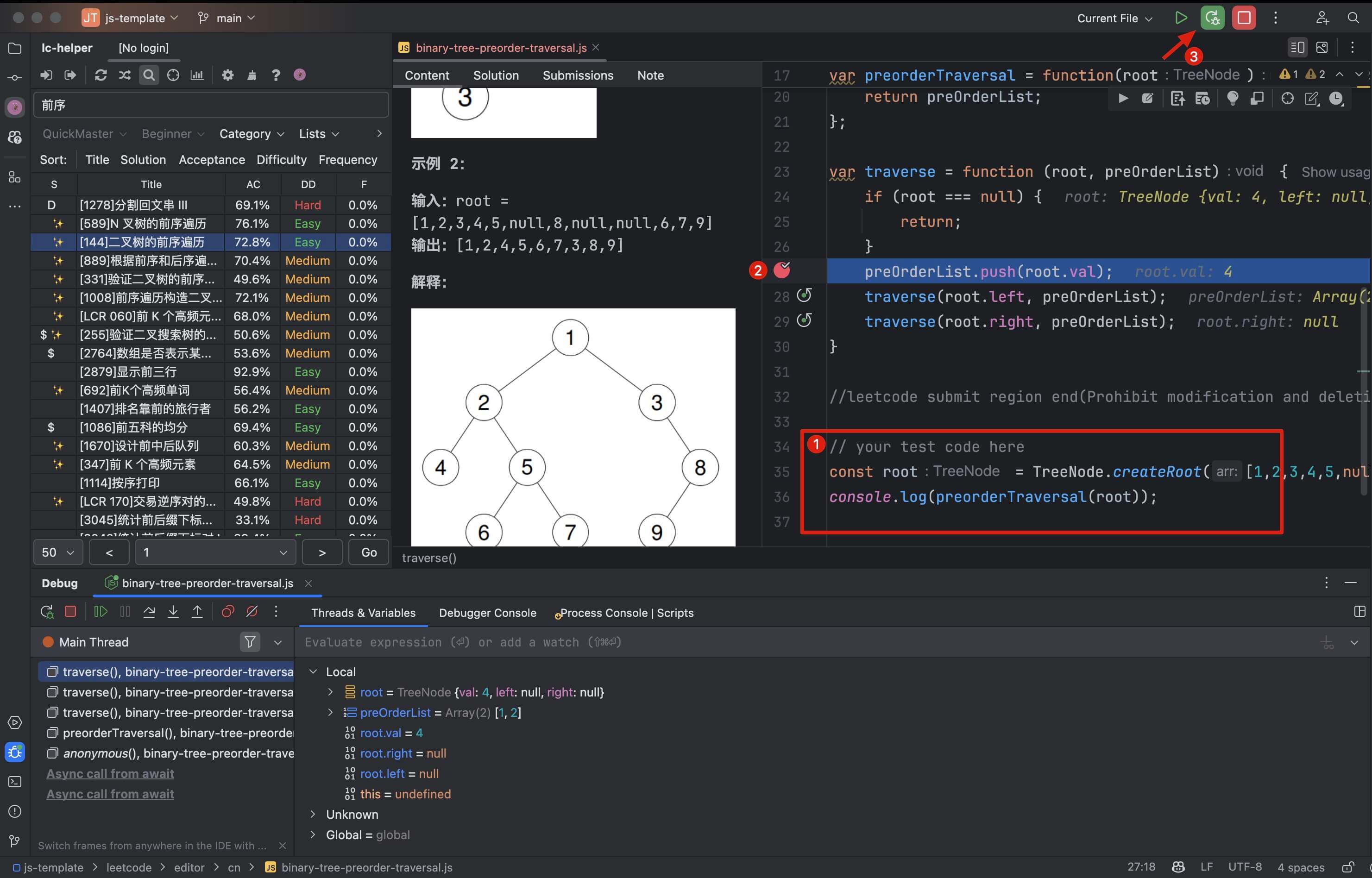
Unlocking Solutions/Question List on This Site
All users can freely access the plugin's problem-solving features and some of this site's solution guides. By purchasing site subscription, you can unlock all solution guides, algorithm visualization panels, and question lists in the plugin.
If you have purchased a subscription, please follow the steps below to unlock the site's solutions in the plugin.
Step 1: Install and Launch the Plugin
Follow the instructions above to install the plugin and log in to your LeetCode account, ensuring the basic functionality of the plugin is working.
Step 2: Obtain Site Cookie
Click the button below to copy the cookie needed to log into the plugin:
Step 3: Manually Refresh Data
After copying the cookie, follow the steps shown in the image below:
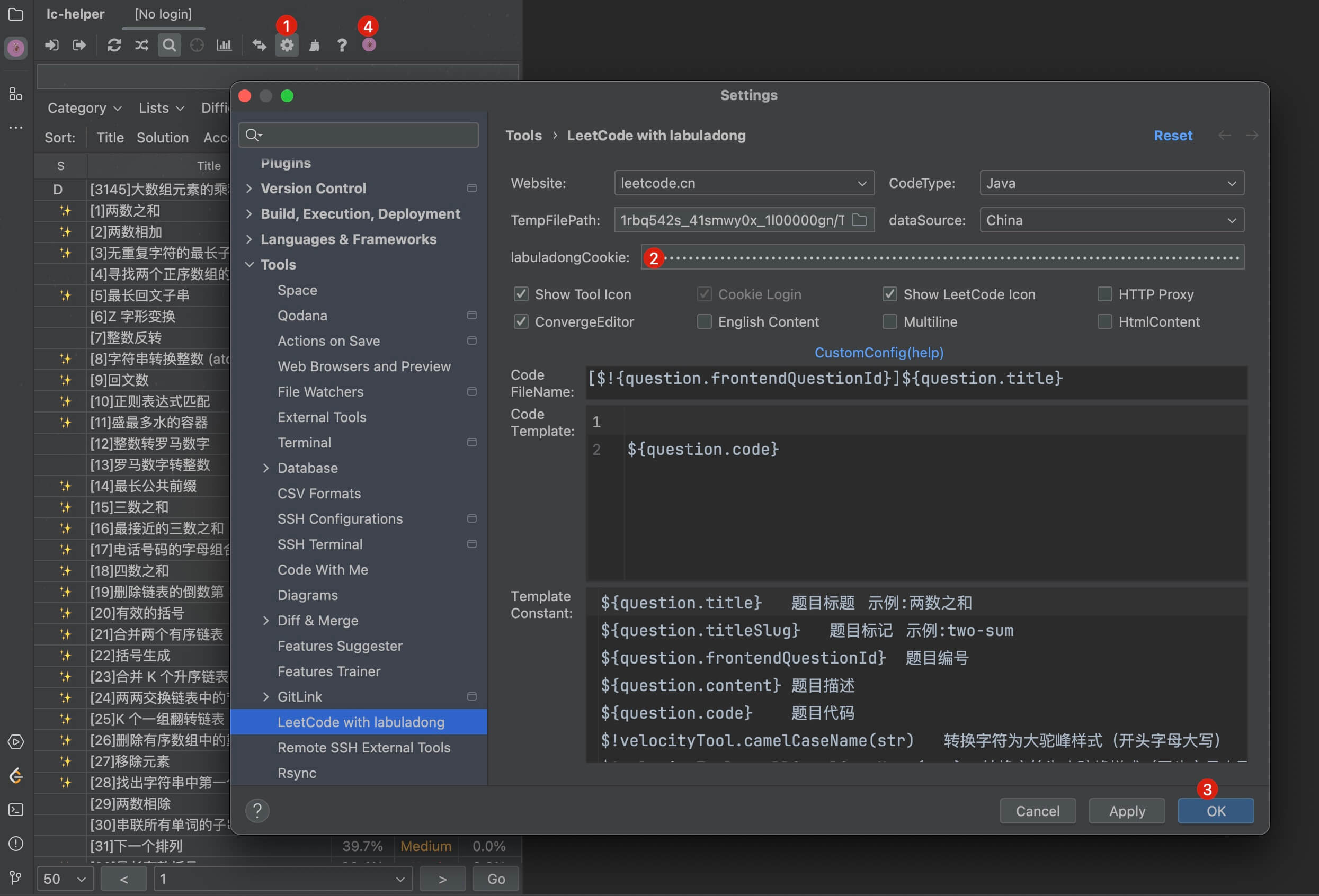
1️⃣ Click the settings icon to enter the settings page.
2️⃣ Paste the cookie into the input field.
3️⃣ Click the OK button.
4️⃣ Click the labuladong website icon to manually trigger data fetching.
The plugin will first fetch some public data, and finally fetch the exclusive solution data for subscribers. If data fetching is slow, try disabling all network proxies or switching networks.
The final output should look similar to the following:
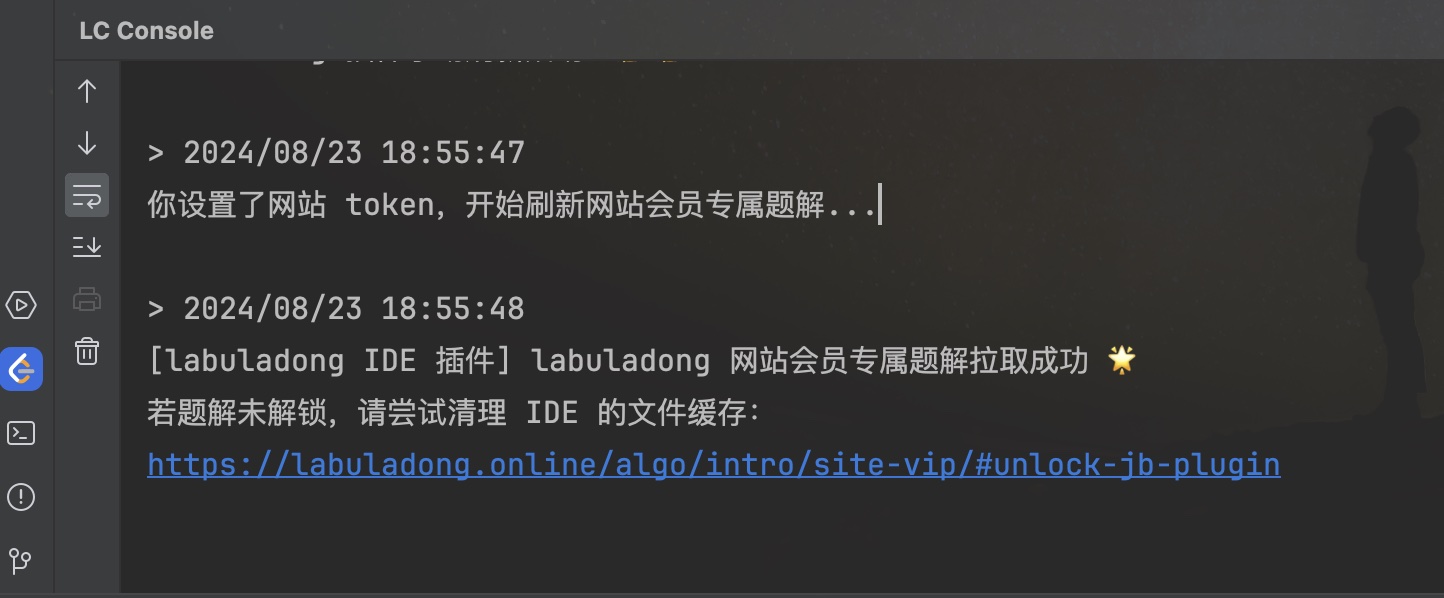
If this output is not visible, there might be a network issue. Try disabling system proxies or switching networks.
Step 4: Clear IDE File Cache
If the data fetch is successful, you should be able to view all solution guides in the plugin. However, due to caching, previously opened questions may still appear locked. This is because the IDE's file system cache has not been refreshed.
Please close all open question files first, then manually clear the IDE's cache and restart the IDE to resolve this:
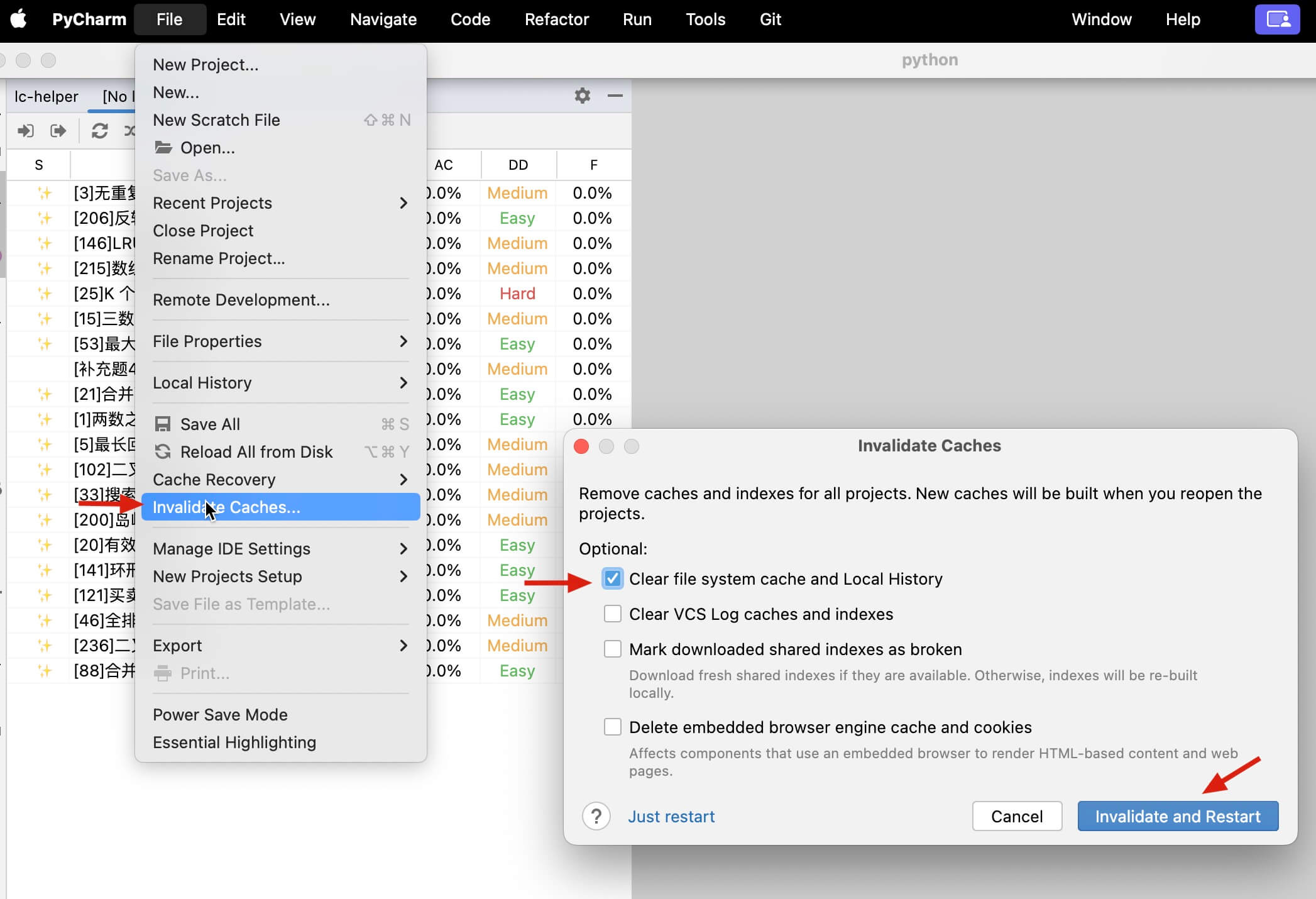
Update Method
JetBrains IDEs automatically detect updates and prompt you when updates are available. It is recommended to update to the latest version promptly to ensure the smoothest experience.
Changelog
For details, refer to the JetBrain Plugin Changelog.
Frequently Asked Questions
How to Debug Code Locally
To utilize the custom code template feature, please carefully refer to the "Local Code Debugging" section in the usage guide above.
Chinese Characters Garbled?
This issue generally does not occur with newer versions of IDEs and operating systems. If it does, you can refer to this post to modify the IDE's encoding to UTF-8.
Problem Page Suddenly Turns Blank?
Has your IDE been installed and used for a long time? Only heavy users of the IDE may encounter this bug. It is difficult to diagnose the cause, but it is likely related to some cache/internal configuration issues during the IDE upgrade process. The simplest solution is to download the latest version of the IDE from the official website and reinstall it locally (don't worry, your existing configurations will not be overwritten), and all problems should disappear.
No ✨ Mark in the Problem List?
If the problem list does not show the ✨ mark after logging in, you can manually refresh the labuladong solution data by clicking the website logo icon on the far right of the toolbar:
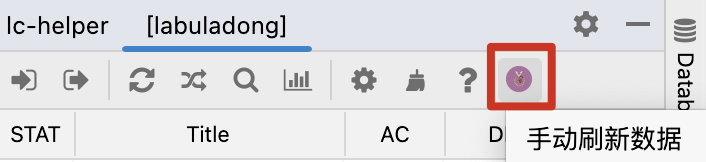
After waiting for about ten seconds, you will see a notification bar indicating "Manual refresh of labuladong data successful," and the ✨ mark will appear.
If there is still no response and no error is reported, it is most likely a network issue. Please try turning off the proxy or changing the network and refresh the data again.
✨ Mark Present but No Solution or Thought Buttons?
If the data retrieval is successful but the problem details page does not show the "labuladong Solution" and "labuladong Thought" buttons, it is because the IDE's file system cache has not been refreshed.
You can try manually clearing the IDE's cache and restarting the IDE:
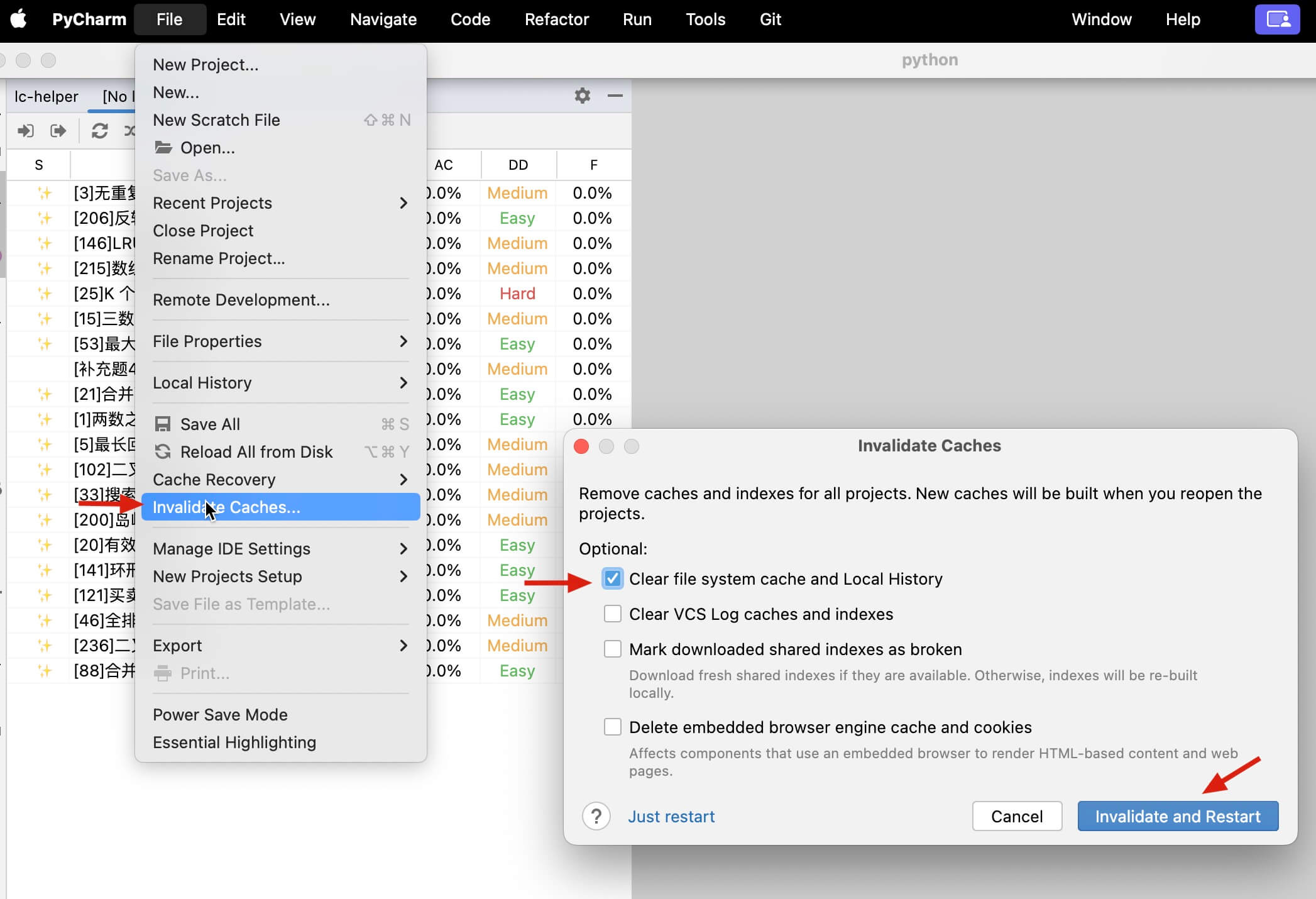
Code Does Not Auto-Complete or Correct?
Code completion and correction are fundamental features of an IDE and are not related to plugins. If these features are not working, it is usually because your IDE is not properly configured or your code file is not recognized by the IDE.
For example, in the Go language, you need to add package main
at the top of your code file for it to be recognized by the Goland IDE. You can use the "Local Debugging Code" feature introduced earlier to automatically add this line.
Similarly, for Java files, they need to be part of a Java project to be recognized by the IDE. You can modify the plugin settings by setting TempFilePath
to your project path and configuring Code Template to automatically add the package name. This way, the code file will be saved in the project, and the IDE will recognize it and provide code completion.
No Code Submission Button?
Older plugin versions might have this issue. Please ensure you are using the latest version of the plugin. Generally, when you move your mouse to the code editing area, buttons for submission, testing, etc., should appear in the upper right corner.
Additionally, right-clicking in the code file will also display options for submission, testing, and other functions.
Bug Reporting
You can create an issue on GitHub to report problems: